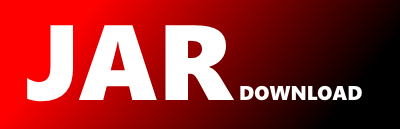
com.pulumi.azurenative.compute.outputs.ImageDataDiskResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute.outputs;
import com.pulumi.azurenative.compute.outputs.DiskEncryptionSetParametersResponse;
import com.pulumi.azurenative.compute.outputs.SubResourceResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ImageDataDiskResponse {
/**
* @return The Virtual Hard Disk.
*
*/
private @Nullable String blobUri;
/**
* @return Specifies the caching requirements. Possible values are: **None,** **ReadOnly,** **ReadWrite.** The default values are: **None for Standard storage. ReadOnly for Premium storage.**
*
*/
private @Nullable String caching;
/**
* @return Specifies the customer managed disk encryption set resource id for the managed image disk.
*
*/
private @Nullable DiskEncryptionSetParametersResponse diskEncryptionSet;
/**
* @return Specifies the size of empty data disks in gigabytes. This element can be used to overwrite the name of the disk in a virtual machine image. This value cannot be larger than 1023 GB.
*
*/
private @Nullable Integer diskSizeGB;
/**
* @return Specifies the logical unit number of the data disk. This value is used to identify data disks within the VM and therefore must be unique for each data disk attached to a VM.
*
*/
private Integer lun;
/**
* @return The managedDisk.
*
*/
private @Nullable SubResourceResponse managedDisk;
/**
* @return The snapshot.
*
*/
private @Nullable SubResourceResponse snapshot;
/**
* @return Specifies the storage account type for the managed disk. NOTE: UltraSSD_LRS can only be used with data disks, it cannot be used with OS Disk.
*
*/
private @Nullable String storageAccountType;
private ImageDataDiskResponse() {}
/**
* @return The Virtual Hard Disk.
*
*/
public Optional blobUri() {
return Optional.ofNullable(this.blobUri);
}
/**
* @return Specifies the caching requirements. Possible values are: **None,** **ReadOnly,** **ReadWrite.** The default values are: **None for Standard storage. ReadOnly for Premium storage.**
*
*/
public Optional caching() {
return Optional.ofNullable(this.caching);
}
/**
* @return Specifies the customer managed disk encryption set resource id for the managed image disk.
*
*/
public Optional diskEncryptionSet() {
return Optional.ofNullable(this.diskEncryptionSet);
}
/**
* @return Specifies the size of empty data disks in gigabytes. This element can be used to overwrite the name of the disk in a virtual machine image. This value cannot be larger than 1023 GB.
*
*/
public Optional diskSizeGB() {
return Optional.ofNullable(this.diskSizeGB);
}
/**
* @return Specifies the logical unit number of the data disk. This value is used to identify data disks within the VM and therefore must be unique for each data disk attached to a VM.
*
*/
public Integer lun() {
return this.lun;
}
/**
* @return The managedDisk.
*
*/
public Optional managedDisk() {
return Optional.ofNullable(this.managedDisk);
}
/**
* @return The snapshot.
*
*/
public Optional snapshot() {
return Optional.ofNullable(this.snapshot);
}
/**
* @return Specifies the storage account type for the managed disk. NOTE: UltraSSD_LRS can only be used with data disks, it cannot be used with OS Disk.
*
*/
public Optional storageAccountType() {
return Optional.ofNullable(this.storageAccountType);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ImageDataDiskResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String blobUri;
private @Nullable String caching;
private @Nullable DiskEncryptionSetParametersResponse diskEncryptionSet;
private @Nullable Integer diskSizeGB;
private Integer lun;
private @Nullable SubResourceResponse managedDisk;
private @Nullable SubResourceResponse snapshot;
private @Nullable String storageAccountType;
public Builder() {}
public Builder(ImageDataDiskResponse defaults) {
Objects.requireNonNull(defaults);
this.blobUri = defaults.blobUri;
this.caching = defaults.caching;
this.diskEncryptionSet = defaults.diskEncryptionSet;
this.diskSizeGB = defaults.diskSizeGB;
this.lun = defaults.lun;
this.managedDisk = defaults.managedDisk;
this.snapshot = defaults.snapshot;
this.storageAccountType = defaults.storageAccountType;
}
@CustomType.Setter
public Builder blobUri(@Nullable String blobUri) {
this.blobUri = blobUri;
return this;
}
@CustomType.Setter
public Builder caching(@Nullable String caching) {
this.caching = caching;
return this;
}
@CustomType.Setter
public Builder diskEncryptionSet(@Nullable DiskEncryptionSetParametersResponse diskEncryptionSet) {
this.diskEncryptionSet = diskEncryptionSet;
return this;
}
@CustomType.Setter
public Builder diskSizeGB(@Nullable Integer diskSizeGB) {
this.diskSizeGB = diskSizeGB;
return this;
}
@CustomType.Setter
public Builder lun(Integer lun) {
if (lun == null) {
throw new MissingRequiredPropertyException("ImageDataDiskResponse", "lun");
}
this.lun = lun;
return this;
}
@CustomType.Setter
public Builder managedDisk(@Nullable SubResourceResponse managedDisk) {
this.managedDisk = managedDisk;
return this;
}
@CustomType.Setter
public Builder snapshot(@Nullable SubResourceResponse snapshot) {
this.snapshot = snapshot;
return this;
}
@CustomType.Setter
public Builder storageAccountType(@Nullable String storageAccountType) {
this.storageAccountType = storageAccountType;
return this;
}
public ImageDataDiskResponse build() {
final var _resultValue = new ImageDataDiskResponse();
_resultValue.blobUri = blobUri;
_resultValue.caching = caching;
_resultValue.diskEncryptionSet = diskEncryptionSet;
_resultValue.diskSizeGB = diskSizeGB;
_resultValue.lun = lun;
_resultValue.managedDisk = managedDisk;
_resultValue.snapshot = snapshot;
_resultValue.storageAccountType = storageAccountType;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy