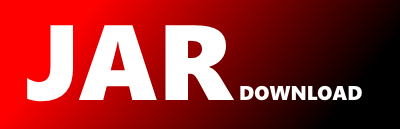
com.pulumi.azurenative.compute.outputs.OSDiskResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute.outputs;
import com.pulumi.azurenative.compute.outputs.DiffDiskSettingsResponse;
import com.pulumi.azurenative.compute.outputs.DiskEncryptionSettingsResponse;
import com.pulumi.azurenative.compute.outputs.ManagedDiskParametersResponse;
import com.pulumi.azurenative.compute.outputs.VirtualHardDiskResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class OSDiskResponse {
/**
* @return Specifies the caching requirements. Possible values are: **None,** **ReadOnly,** **ReadWrite.** The defaulting behavior is: **None for Standard storage. ReadOnly for Premium storage.**
*
*/
private @Nullable String caching;
/**
* @return Specifies how the virtual machine should be created. Possible values are: **Attach.** This value is used when you are using a specialized disk to create the virtual machine. **FromImage.** This value is used when you are using an image to create the virtual machine. If you are using a platform image, you should also use the imageReference element described above. If you are using a marketplace image, you should also use the plan element previously described.
*
*/
private String createOption;
/**
* @return Specifies whether OS Disk should be deleted or detached upon VM deletion. Possible values are: **Delete.** If this value is used, the OS disk is deleted when VM is deleted. **Detach.** If this value is used, the os disk is retained after VM is deleted. The default value is set to **Detach**. For an ephemeral OS Disk, the default value is set to **Delete**. The user cannot change the delete option for an ephemeral OS Disk.
*
*/
private @Nullable String deleteOption;
/**
* @return Specifies the ephemeral Disk Settings for the operating system disk used by the virtual machine.
*
*/
private @Nullable DiffDiskSettingsResponse diffDiskSettings;
/**
* @return Specifies the size of an empty data disk in gigabytes. This element can be used to overwrite the size of the disk in a virtual machine image. The property 'diskSizeGB' is the number of bytes x 1024^3 for the disk and the value cannot be larger than 1023.
*
*/
private @Nullable Integer diskSizeGB;
/**
* @return Specifies the encryption settings for the OS Disk. Minimum api-version: 2015-06-15.
*
*/
private @Nullable DiskEncryptionSettingsResponse encryptionSettings;
/**
* @return The source user image virtual hard disk. The virtual hard disk will be copied before being attached to the virtual machine. If SourceImage is provided, the destination virtual hard drive must not exist.
*
*/
private @Nullable VirtualHardDiskResponse image;
/**
* @return The managed disk parameters.
*
*/
private @Nullable ManagedDiskParametersResponse managedDisk;
/**
* @return The disk name.
*
*/
private @Nullable String name;
/**
* @return This property allows you to specify the type of the OS that is included in the disk if creating a VM from user-image or a specialized VHD. Possible values are: **Windows,** **Linux.**
*
*/
private @Nullable String osType;
/**
* @return The virtual hard disk.
*
*/
private @Nullable VirtualHardDiskResponse vhd;
/**
* @return Specifies whether writeAccelerator should be enabled or disabled on the disk.
*
*/
private @Nullable Boolean writeAcceleratorEnabled;
private OSDiskResponse() {}
/**
* @return Specifies the caching requirements. Possible values are: **None,** **ReadOnly,** **ReadWrite.** The defaulting behavior is: **None for Standard storage. ReadOnly for Premium storage.**
*
*/
public Optional caching() {
return Optional.ofNullable(this.caching);
}
/**
* @return Specifies how the virtual machine should be created. Possible values are: **Attach.** This value is used when you are using a specialized disk to create the virtual machine. **FromImage.** This value is used when you are using an image to create the virtual machine. If you are using a platform image, you should also use the imageReference element described above. If you are using a marketplace image, you should also use the plan element previously described.
*
*/
public String createOption() {
return this.createOption;
}
/**
* @return Specifies whether OS Disk should be deleted or detached upon VM deletion. Possible values are: **Delete.** If this value is used, the OS disk is deleted when VM is deleted. **Detach.** If this value is used, the os disk is retained after VM is deleted. The default value is set to **Detach**. For an ephemeral OS Disk, the default value is set to **Delete**. The user cannot change the delete option for an ephemeral OS Disk.
*
*/
public Optional deleteOption() {
return Optional.ofNullable(this.deleteOption);
}
/**
* @return Specifies the ephemeral Disk Settings for the operating system disk used by the virtual machine.
*
*/
public Optional diffDiskSettings() {
return Optional.ofNullable(this.diffDiskSettings);
}
/**
* @return Specifies the size of an empty data disk in gigabytes. This element can be used to overwrite the size of the disk in a virtual machine image. The property 'diskSizeGB' is the number of bytes x 1024^3 for the disk and the value cannot be larger than 1023.
*
*/
public Optional diskSizeGB() {
return Optional.ofNullable(this.diskSizeGB);
}
/**
* @return Specifies the encryption settings for the OS Disk. Minimum api-version: 2015-06-15.
*
*/
public Optional encryptionSettings() {
return Optional.ofNullable(this.encryptionSettings);
}
/**
* @return The source user image virtual hard disk. The virtual hard disk will be copied before being attached to the virtual machine. If SourceImage is provided, the destination virtual hard drive must not exist.
*
*/
public Optional image() {
return Optional.ofNullable(this.image);
}
/**
* @return The managed disk parameters.
*
*/
public Optional managedDisk() {
return Optional.ofNullable(this.managedDisk);
}
/**
* @return The disk name.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return This property allows you to specify the type of the OS that is included in the disk if creating a VM from user-image or a specialized VHD. Possible values are: **Windows,** **Linux.**
*
*/
public Optional osType() {
return Optional.ofNullable(this.osType);
}
/**
* @return The virtual hard disk.
*
*/
public Optional vhd() {
return Optional.ofNullable(this.vhd);
}
/**
* @return Specifies whether writeAccelerator should be enabled or disabled on the disk.
*
*/
public Optional writeAcceleratorEnabled() {
return Optional.ofNullable(this.writeAcceleratorEnabled);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(OSDiskResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String caching;
private String createOption;
private @Nullable String deleteOption;
private @Nullable DiffDiskSettingsResponse diffDiskSettings;
private @Nullable Integer diskSizeGB;
private @Nullable DiskEncryptionSettingsResponse encryptionSettings;
private @Nullable VirtualHardDiskResponse image;
private @Nullable ManagedDiskParametersResponse managedDisk;
private @Nullable String name;
private @Nullable String osType;
private @Nullable VirtualHardDiskResponse vhd;
private @Nullable Boolean writeAcceleratorEnabled;
public Builder() {}
public Builder(OSDiskResponse defaults) {
Objects.requireNonNull(defaults);
this.caching = defaults.caching;
this.createOption = defaults.createOption;
this.deleteOption = defaults.deleteOption;
this.diffDiskSettings = defaults.diffDiskSettings;
this.diskSizeGB = defaults.diskSizeGB;
this.encryptionSettings = defaults.encryptionSettings;
this.image = defaults.image;
this.managedDisk = defaults.managedDisk;
this.name = defaults.name;
this.osType = defaults.osType;
this.vhd = defaults.vhd;
this.writeAcceleratorEnabled = defaults.writeAcceleratorEnabled;
}
@CustomType.Setter
public Builder caching(@Nullable String caching) {
this.caching = caching;
return this;
}
@CustomType.Setter
public Builder createOption(String createOption) {
if (createOption == null) {
throw new MissingRequiredPropertyException("OSDiskResponse", "createOption");
}
this.createOption = createOption;
return this;
}
@CustomType.Setter
public Builder deleteOption(@Nullable String deleteOption) {
this.deleteOption = deleteOption;
return this;
}
@CustomType.Setter
public Builder diffDiskSettings(@Nullable DiffDiskSettingsResponse diffDiskSettings) {
this.diffDiskSettings = diffDiskSettings;
return this;
}
@CustomType.Setter
public Builder diskSizeGB(@Nullable Integer diskSizeGB) {
this.diskSizeGB = diskSizeGB;
return this;
}
@CustomType.Setter
public Builder encryptionSettings(@Nullable DiskEncryptionSettingsResponse encryptionSettings) {
this.encryptionSettings = encryptionSettings;
return this;
}
@CustomType.Setter
public Builder image(@Nullable VirtualHardDiskResponse image) {
this.image = image;
return this;
}
@CustomType.Setter
public Builder managedDisk(@Nullable ManagedDiskParametersResponse managedDisk) {
this.managedDisk = managedDisk;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder osType(@Nullable String osType) {
this.osType = osType;
return this;
}
@CustomType.Setter
public Builder vhd(@Nullable VirtualHardDiskResponse vhd) {
this.vhd = vhd;
return this;
}
@CustomType.Setter
public Builder writeAcceleratorEnabled(@Nullable Boolean writeAcceleratorEnabled) {
this.writeAcceleratorEnabled = writeAcceleratorEnabled;
return this;
}
public OSDiskResponse build() {
final var _resultValue = new OSDiskResponse();
_resultValue.caching = caching;
_resultValue.createOption = createOption;
_resultValue.deleteOption = deleteOption;
_resultValue.diffDiskSettings = diffDiskSettings;
_resultValue.diskSizeGB = diskSizeGB;
_resultValue.encryptionSettings = encryptionSettings;
_resultValue.image = image;
_resultValue.managedDisk = managedDisk;
_resultValue.name = name;
_resultValue.osType = osType;
_resultValue.vhd = vhd;
_resultValue.writeAcceleratorEnabled = writeAcceleratorEnabled;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy