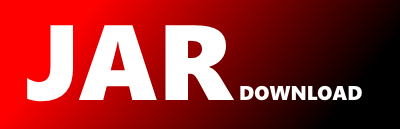
com.pulumi.azurenative.connectedcache.outputs.AdditionalCustomerPropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy