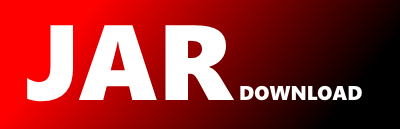
com.pulumi.azurenative.containerinstance.ContainerGroupProfileArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.containerinstance;
import com.pulumi.azurenative.containerinstance.enums.ContainerGroupPriority;
import com.pulumi.azurenative.containerinstance.enums.ContainerGroupRestartPolicy;
import com.pulumi.azurenative.containerinstance.enums.ContainerGroupSku;
import com.pulumi.azurenative.containerinstance.enums.OperatingSystemTypes;
import com.pulumi.azurenative.containerinstance.inputs.ConfidentialComputePropertiesArgs;
import com.pulumi.azurenative.containerinstance.inputs.ContainerArgs;
import com.pulumi.azurenative.containerinstance.inputs.ContainerGroupDiagnosticsArgs;
import com.pulumi.azurenative.containerinstance.inputs.DeploymentExtensionSpecArgs;
import com.pulumi.azurenative.containerinstance.inputs.EncryptionPropertiesArgs;
import com.pulumi.azurenative.containerinstance.inputs.ImageRegistryCredentialArgs;
import com.pulumi.azurenative.containerinstance.inputs.InitContainerDefinitionArgs;
import com.pulumi.azurenative.containerinstance.inputs.IpAddressArgs;
import com.pulumi.azurenative.containerinstance.inputs.VolumeArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ContainerGroupProfileArgs extends com.pulumi.resources.ResourceArgs {
public static final ContainerGroupProfileArgs Empty = new ContainerGroupProfileArgs();
/**
* The properties for confidential container group
*
*/
@Import(name="confidentialComputeProperties")
private @Nullable Output confidentialComputeProperties;
/**
* @return The properties for confidential container group
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy