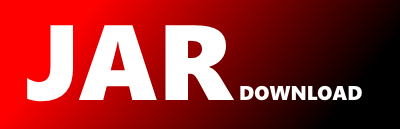
com.pulumi.azurenative.containerregistry.outputs.EncodedTaskRunRequestResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.containerregistry.outputs;
import com.pulumi.azurenative.containerregistry.outputs.AgentPropertiesResponse;
import com.pulumi.azurenative.containerregistry.outputs.CredentialsResponse;
import com.pulumi.azurenative.containerregistry.outputs.PlatformPropertiesResponse;
import com.pulumi.azurenative.containerregistry.outputs.SetValueResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class EncodedTaskRunRequestResponse {
/**
* @return The machine configuration of the run agent.
*
*/
private @Nullable AgentPropertiesResponse agentConfiguration;
/**
* @return The dedicated agent pool for the run.
*
*/
private @Nullable String agentPoolName;
/**
* @return The properties that describes a set of credentials that will be used when this run is invoked.
*
*/
private @Nullable CredentialsResponse credentials;
/**
* @return Base64 encoded value of the template/definition file content.
*
*/
private String encodedTaskContent;
/**
* @return Base64 encoded value of the parameters/values file content.
*
*/
private @Nullable String encodedValuesContent;
/**
* @return The value that indicates whether archiving is enabled for the run or not.
*
*/
private @Nullable Boolean isArchiveEnabled;
/**
* @return The template that describes the repository and tag information for run log artifact.
*
*/
private @Nullable String logTemplate;
/**
* @return The platform properties against which the run has to happen.
*
*/
private PlatformPropertiesResponse platform;
/**
* @return The URL(absolute or relative) of the source context. It can be an URL to a tar or git repository.
* If it is relative URL, the relative path should be obtained from calling listBuildSourceUploadUrl API.
*
*/
private @Nullable String sourceLocation;
/**
* @return Run timeout in seconds.
*
*/
private @Nullable Integer timeout;
/**
* @return The type of the run request.
* Expected value is 'EncodedTaskRunRequest'.
*
*/
private String type;
/**
* @return The collection of overridable values that can be passed when running a task.
*
*/
private @Nullable List values;
private EncodedTaskRunRequestResponse() {}
/**
* @return The machine configuration of the run agent.
*
*/
public Optional agentConfiguration() {
return Optional.ofNullable(this.agentConfiguration);
}
/**
* @return The dedicated agent pool for the run.
*
*/
public Optional agentPoolName() {
return Optional.ofNullable(this.agentPoolName);
}
/**
* @return The properties that describes a set of credentials that will be used when this run is invoked.
*
*/
public Optional credentials() {
return Optional.ofNullable(this.credentials);
}
/**
* @return Base64 encoded value of the template/definition file content.
*
*/
public String encodedTaskContent() {
return this.encodedTaskContent;
}
/**
* @return Base64 encoded value of the parameters/values file content.
*
*/
public Optional encodedValuesContent() {
return Optional.ofNullable(this.encodedValuesContent);
}
/**
* @return The value that indicates whether archiving is enabled for the run or not.
*
*/
public Optional isArchiveEnabled() {
return Optional.ofNullable(this.isArchiveEnabled);
}
/**
* @return The template that describes the repository and tag information for run log artifact.
*
*/
public Optional logTemplate() {
return Optional.ofNullable(this.logTemplate);
}
/**
* @return The platform properties against which the run has to happen.
*
*/
public PlatformPropertiesResponse platform() {
return this.platform;
}
/**
* @return The URL(absolute or relative) of the source context. It can be an URL to a tar or git repository.
* If it is relative URL, the relative path should be obtained from calling listBuildSourceUploadUrl API.
*
*/
public Optional sourceLocation() {
return Optional.ofNullable(this.sourceLocation);
}
/**
* @return Run timeout in seconds.
*
*/
public Optional timeout() {
return Optional.ofNullable(this.timeout);
}
/**
* @return The type of the run request.
* Expected value is 'EncodedTaskRunRequest'.
*
*/
public String type() {
return this.type;
}
/**
* @return The collection of overridable values that can be passed when running a task.
*
*/
public List values() {
return this.values == null ? List.of() : this.values;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(EncodedTaskRunRequestResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable AgentPropertiesResponse agentConfiguration;
private @Nullable String agentPoolName;
private @Nullable CredentialsResponse credentials;
private String encodedTaskContent;
private @Nullable String encodedValuesContent;
private @Nullable Boolean isArchiveEnabled;
private @Nullable String logTemplate;
private PlatformPropertiesResponse platform;
private @Nullable String sourceLocation;
private @Nullable Integer timeout;
private String type;
private @Nullable List values;
public Builder() {}
public Builder(EncodedTaskRunRequestResponse defaults) {
Objects.requireNonNull(defaults);
this.agentConfiguration = defaults.agentConfiguration;
this.agentPoolName = defaults.agentPoolName;
this.credentials = defaults.credentials;
this.encodedTaskContent = defaults.encodedTaskContent;
this.encodedValuesContent = defaults.encodedValuesContent;
this.isArchiveEnabled = defaults.isArchiveEnabled;
this.logTemplate = defaults.logTemplate;
this.platform = defaults.platform;
this.sourceLocation = defaults.sourceLocation;
this.timeout = defaults.timeout;
this.type = defaults.type;
this.values = defaults.values;
}
@CustomType.Setter
public Builder agentConfiguration(@Nullable AgentPropertiesResponse agentConfiguration) {
this.agentConfiguration = agentConfiguration;
return this;
}
@CustomType.Setter
public Builder agentPoolName(@Nullable String agentPoolName) {
this.agentPoolName = agentPoolName;
return this;
}
@CustomType.Setter
public Builder credentials(@Nullable CredentialsResponse credentials) {
this.credentials = credentials;
return this;
}
@CustomType.Setter
public Builder encodedTaskContent(String encodedTaskContent) {
if (encodedTaskContent == null) {
throw new MissingRequiredPropertyException("EncodedTaskRunRequestResponse", "encodedTaskContent");
}
this.encodedTaskContent = encodedTaskContent;
return this;
}
@CustomType.Setter
public Builder encodedValuesContent(@Nullable String encodedValuesContent) {
this.encodedValuesContent = encodedValuesContent;
return this;
}
@CustomType.Setter
public Builder isArchiveEnabled(@Nullable Boolean isArchiveEnabled) {
this.isArchiveEnabled = isArchiveEnabled;
return this;
}
@CustomType.Setter
public Builder logTemplate(@Nullable String logTemplate) {
this.logTemplate = logTemplate;
return this;
}
@CustomType.Setter
public Builder platform(PlatformPropertiesResponse platform) {
if (platform == null) {
throw new MissingRequiredPropertyException("EncodedTaskRunRequestResponse", "platform");
}
this.platform = platform;
return this;
}
@CustomType.Setter
public Builder sourceLocation(@Nullable String sourceLocation) {
this.sourceLocation = sourceLocation;
return this;
}
@CustomType.Setter
public Builder timeout(@Nullable Integer timeout) {
this.timeout = timeout;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("EncodedTaskRunRequestResponse", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder values(@Nullable List values) {
this.values = values;
return this;
}
public Builder values(SetValueResponse... values) {
return values(List.of(values));
}
public EncodedTaskRunRequestResponse build() {
final var _resultValue = new EncodedTaskRunRequestResponse();
_resultValue.agentConfiguration = agentConfiguration;
_resultValue.agentPoolName = agentPoolName;
_resultValue.credentials = credentials;
_resultValue.encodedTaskContent = encodedTaskContent;
_resultValue.encodedValuesContent = encodedValuesContent;
_resultValue.isArchiveEnabled = isArchiveEnabled;
_resultValue.logTemplate = logTemplate;
_resultValue.platform = platform;
_resultValue.sourceLocation = sourceLocation;
_resultValue.timeout = timeout;
_resultValue.type = type;
_resultValue.values = values;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy