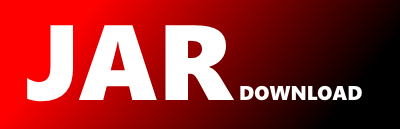
com.pulumi.azurenative.customerinsights.ProfileArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.customerinsights;
import com.pulumi.azurenative.customerinsights.enums.EntityTypes;
import com.pulumi.azurenative.customerinsights.inputs.PropertyDefinitionArgs;
import com.pulumi.azurenative.customerinsights.inputs.StrongIdArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ProfileArgs extends com.pulumi.resources.ResourceArgs {
public static final ProfileArgs Empty = new ProfileArgs();
/**
* The api entity set name. This becomes the odata entity set name for the entity Type being referred in this object.
*
*/
@Import(name="apiEntitySetName")
private @Nullable Output apiEntitySetName;
/**
* @return The api entity set name. This becomes the odata entity set name for the entity Type being referred in this object.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy