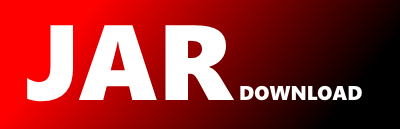
com.pulumi.azurenative.customerinsights.RoleAssignmentArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.customerinsights;
import com.pulumi.azurenative.customerinsights.enums.RoleTypes;
import com.pulumi.azurenative.customerinsights.inputs.AssignmentPrincipalArgs;
import com.pulumi.azurenative.customerinsights.inputs.ResourceSetDescriptionArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class RoleAssignmentArgs extends com.pulumi.resources.ResourceArgs {
public static final RoleAssignmentArgs Empty = new RoleAssignmentArgs();
/**
* The assignment name
*
*/
@Import(name="assignmentName")
private @Nullable Output assignmentName;
/**
* @return The assignment name
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy