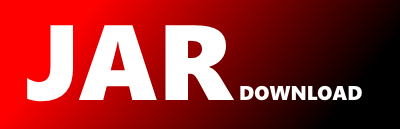
com.pulumi.azurenative.databasewatcher.outputs.DatastoreResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.databasewatcher.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class DatastoreResponse {
/**
* @return The Azure ResourceId of an Azure Data Explorer cluster.
*
*/
private @Nullable String adxClusterResourceId;
/**
* @return The Kusto cluster display name.
*
*/
private @Nullable String kustoClusterDisplayName;
/**
* @return The Kusto cluster URI.
*
*/
private String kustoClusterUri;
/**
* @return The Kusto data ingestion URI.
*
*/
private String kustoDataIngestionUri;
/**
* @return The name of a Kusto database.
*
*/
private String kustoDatabaseName;
/**
* @return The Kusto management URL.
*
*/
private String kustoManagementUrl;
/**
* @return The type of a Kusto offering.
*
*/
private String kustoOfferingType;
private DatastoreResponse() {}
/**
* @return The Azure ResourceId of an Azure Data Explorer cluster.
*
*/
public Optional adxClusterResourceId() {
return Optional.ofNullable(this.adxClusterResourceId);
}
/**
* @return The Kusto cluster display name.
*
*/
public Optional kustoClusterDisplayName() {
return Optional.ofNullable(this.kustoClusterDisplayName);
}
/**
* @return The Kusto cluster URI.
*
*/
public String kustoClusterUri() {
return this.kustoClusterUri;
}
/**
* @return The Kusto data ingestion URI.
*
*/
public String kustoDataIngestionUri() {
return this.kustoDataIngestionUri;
}
/**
* @return The name of a Kusto database.
*
*/
public String kustoDatabaseName() {
return this.kustoDatabaseName;
}
/**
* @return The Kusto management URL.
*
*/
public String kustoManagementUrl() {
return this.kustoManagementUrl;
}
/**
* @return The type of a Kusto offering.
*
*/
public String kustoOfferingType() {
return this.kustoOfferingType;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(DatastoreResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String adxClusterResourceId;
private @Nullable String kustoClusterDisplayName;
private String kustoClusterUri;
private String kustoDataIngestionUri;
private String kustoDatabaseName;
private String kustoManagementUrl;
private String kustoOfferingType;
public Builder() {}
public Builder(DatastoreResponse defaults) {
Objects.requireNonNull(defaults);
this.adxClusterResourceId = defaults.adxClusterResourceId;
this.kustoClusterDisplayName = defaults.kustoClusterDisplayName;
this.kustoClusterUri = defaults.kustoClusterUri;
this.kustoDataIngestionUri = defaults.kustoDataIngestionUri;
this.kustoDatabaseName = defaults.kustoDatabaseName;
this.kustoManagementUrl = defaults.kustoManagementUrl;
this.kustoOfferingType = defaults.kustoOfferingType;
}
@CustomType.Setter
public Builder adxClusterResourceId(@Nullable String adxClusterResourceId) {
this.adxClusterResourceId = adxClusterResourceId;
return this;
}
@CustomType.Setter
public Builder kustoClusterDisplayName(@Nullable String kustoClusterDisplayName) {
this.kustoClusterDisplayName = kustoClusterDisplayName;
return this;
}
@CustomType.Setter
public Builder kustoClusterUri(String kustoClusterUri) {
if (kustoClusterUri == null) {
throw new MissingRequiredPropertyException("DatastoreResponse", "kustoClusterUri");
}
this.kustoClusterUri = kustoClusterUri;
return this;
}
@CustomType.Setter
public Builder kustoDataIngestionUri(String kustoDataIngestionUri) {
if (kustoDataIngestionUri == null) {
throw new MissingRequiredPropertyException("DatastoreResponse", "kustoDataIngestionUri");
}
this.kustoDataIngestionUri = kustoDataIngestionUri;
return this;
}
@CustomType.Setter
public Builder kustoDatabaseName(String kustoDatabaseName) {
if (kustoDatabaseName == null) {
throw new MissingRequiredPropertyException("DatastoreResponse", "kustoDatabaseName");
}
this.kustoDatabaseName = kustoDatabaseName;
return this;
}
@CustomType.Setter
public Builder kustoManagementUrl(String kustoManagementUrl) {
if (kustoManagementUrl == null) {
throw new MissingRequiredPropertyException("DatastoreResponse", "kustoManagementUrl");
}
this.kustoManagementUrl = kustoManagementUrl;
return this;
}
@CustomType.Setter
public Builder kustoOfferingType(String kustoOfferingType) {
if (kustoOfferingType == null) {
throw new MissingRequiredPropertyException("DatastoreResponse", "kustoOfferingType");
}
this.kustoOfferingType = kustoOfferingType;
return this;
}
public DatastoreResponse build() {
final var _resultValue = new DatastoreResponse();
_resultValue.adxClusterResourceId = adxClusterResourceId;
_resultValue.kustoClusterDisplayName = kustoClusterDisplayName;
_resultValue.kustoClusterUri = kustoClusterUri;
_resultValue.kustoDataIngestionUri = kustoDataIngestionUri;
_resultValue.kustoDatabaseName = kustoDatabaseName;
_resultValue.kustoManagementUrl = kustoManagementUrl;
_resultValue.kustoOfferingType = kustoOfferingType;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy