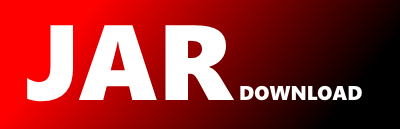
com.pulumi.azurenative.databoxedge.ShareArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.databoxedge;
import com.pulumi.azurenative.databoxedge.enums.DataPolicy;
import com.pulumi.azurenative.databoxedge.enums.MonitoringStatus;
import com.pulumi.azurenative.databoxedge.enums.ShareAccessProtocol;
import com.pulumi.azurenative.databoxedge.enums.ShareStatus;
import com.pulumi.azurenative.databoxedge.inputs.AzureContainerInfoArgs;
import com.pulumi.azurenative.databoxedge.inputs.ClientAccessRightArgs;
import com.pulumi.azurenative.databoxedge.inputs.RefreshDetailsArgs;
import com.pulumi.azurenative.databoxedge.inputs.UserAccessRightArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ShareArgs extends com.pulumi.resources.ResourceArgs {
public static final ShareArgs Empty = new ShareArgs();
/**
* Access protocol to be used by the share.
*
*/
@Import(name="accessProtocol", required=true)
private Output> accessProtocol;
/**
* @return Access protocol to be used by the share.
*
*/
public Output> accessProtocol() {
return this.accessProtocol;
}
/**
* Azure container mapping for the share.
*
*/
@Import(name="azureContainerInfo")
private @Nullable Output azureContainerInfo;
/**
* @return Azure container mapping for the share.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy