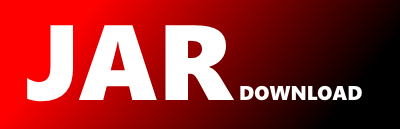
com.pulumi.azurenative.databricks.WorkspaceArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.databricks;
import com.pulumi.azurenative.databricks.enums.PublicNetworkAccess;
import com.pulumi.azurenative.databricks.enums.RequiredNsgRules;
import com.pulumi.azurenative.databricks.inputs.SkuArgs;
import com.pulumi.azurenative.databricks.inputs.WorkspaceCustomParametersArgs;
import com.pulumi.azurenative.databricks.inputs.WorkspacePropertiesEncryptionArgs;
import com.pulumi.azurenative.databricks.inputs.WorkspaceProviderAuthorizationArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class WorkspaceArgs extends com.pulumi.resources.ResourceArgs {
public static final WorkspaceArgs Empty = new WorkspaceArgs();
/**
* The workspace provider authorizations.
*
*/
@Import(name="authorizations")
private @Nullable Output> authorizations;
/**
* @return The workspace provider authorizations.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy