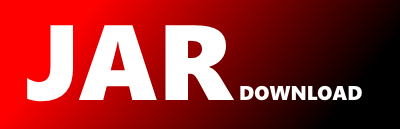
com.pulumi.azurenative.datafactory.ChangeDataCaptureArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datafactory;
import com.pulumi.azurenative.datafactory.inputs.ChangeDataCaptureFolderArgs;
import com.pulumi.azurenative.datafactory.inputs.MapperPolicyArgs;
import com.pulumi.azurenative.datafactory.inputs.MapperSourceConnectionsInfoArgs;
import com.pulumi.azurenative.datafactory.inputs.MapperTargetConnectionsInfoArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ChangeDataCaptureArgs extends com.pulumi.resources.ResourceArgs {
public static final ChangeDataCaptureArgs Empty = new ChangeDataCaptureArgs();
/**
* A boolean to determine if the vnet configuration needs to be overwritten.
*
*/
@Import(name="allowVNetOverride")
private @Nullable Output allowVNetOverride;
/**
* @return A boolean to determine if the vnet configuration needs to be overwritten.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy