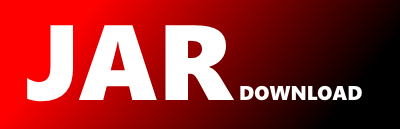
com.pulumi.azurenative.datafactory.inputs.ExecuteWranglingDataflowActivityArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datafactory.inputs;
import com.pulumi.azurenative.datafactory.enums.ActivityOnInactiveMarkAs;
import com.pulumi.azurenative.datafactory.enums.ActivityState;
import com.pulumi.azurenative.datafactory.inputs.ActivityDependencyArgs;
import com.pulumi.azurenative.datafactory.inputs.ActivityPolicyArgs;
import com.pulumi.azurenative.datafactory.inputs.ContinuationSettingsReferenceArgs;
import com.pulumi.azurenative.datafactory.inputs.DataFlowReferenceArgs;
import com.pulumi.azurenative.datafactory.inputs.DataFlowStagingInfoArgs;
import com.pulumi.azurenative.datafactory.inputs.ExecuteDataFlowActivityTypePropertiesComputeArgs;
import com.pulumi.azurenative.datafactory.inputs.IntegrationRuntimeReferenceArgs;
import com.pulumi.azurenative.datafactory.inputs.PowerQuerySinkArgs;
import com.pulumi.azurenative.datafactory.inputs.PowerQuerySinkMappingArgs;
import com.pulumi.azurenative.datafactory.inputs.UserPropertyArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Execute power query activity.
*
*/
public final class ExecuteWranglingDataflowActivityArgs extends com.pulumi.resources.ResourceArgs {
public static final ExecuteWranglingDataflowActivityArgs Empty = new ExecuteWranglingDataflowActivityArgs();
/**
* Compute properties for data flow activity.
*
*/
@Import(name="compute")
private @Nullable Output compute;
/**
* @return Compute properties for data flow activity.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy