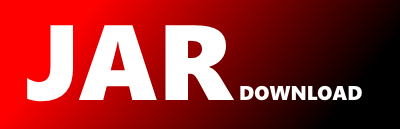
com.pulumi.azurenative.datafactory.outputs.ActivityPolicyResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datafactory.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ActivityPolicyResponse {
/**
* @return Maximum ordinary retry attempts. Default is 0. Type: integer (or Expression with resultType integer), minimum: 0.
*
*/
private @Nullable Object retry;
/**
* @return Interval between each retry attempt (in seconds). The default is 30 sec.
*
*/
private @Nullable Integer retryIntervalInSeconds;
/**
* @return When set to true, Input from activity is considered as secure and will not be logged to monitoring.
*
*/
private @Nullable Boolean secureInput;
/**
* @return When set to true, Output from activity is considered as secure and will not be logged to monitoring.
*
*/
private @Nullable Boolean secureOutput;
/**
* @return Specifies the timeout for the activity to run. The default timeout is 7 days. Type: string (or Expression with resultType string), pattern: ((\d+)\.)?(\d\d):(60|([0-5][0-9])):(60|([0-5][0-9])).
*
*/
private @Nullable Object timeout;
private ActivityPolicyResponse() {}
/**
* @return Maximum ordinary retry attempts. Default is 0. Type: integer (or Expression with resultType integer), minimum: 0.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy