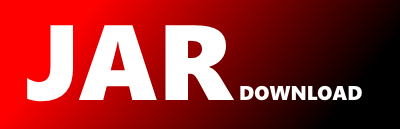
com.pulumi.azurenative.datafactory.outputs.AmazonRedshiftSourceResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datafactory.outputs;
import com.pulumi.azurenative.datafactory.outputs.RedshiftUnloadSettingsResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Object;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class AmazonRedshiftSourceResponse {
/**
* @return Specifies the additional columns to be added to source data. Type: array of objects(AdditionalColumns) (or Expression with resultType array of objects).
*
*/
private @Nullable Object additionalColumns;
/**
* @return If true, disable data store metrics collection. Default is false. Type: boolean (or Expression with resultType boolean).
*
*/
private @Nullable Object disableMetricsCollection;
/**
* @return The maximum concurrent connection count for the source data store. Type: integer (or Expression with resultType integer).
*
*/
private @Nullable Object maxConcurrentConnections;
/**
* @return Database query. Type: string (or Expression with resultType string).
*
*/
private @Nullable Object query;
/**
* @return Query timeout. Type: string (or Expression with resultType string), pattern: ((\d+)\.)?(\d\d):(60|([0-5][0-9])):(60|([0-5][0-9])).
*
*/
private @Nullable Object queryTimeout;
/**
* @return The Amazon S3 settings needed for the interim Amazon S3 when copying from Amazon Redshift with unload. With this, data from Amazon Redshift source will be unloaded into S3 first and then copied into the targeted sink from the interim S3.
*
*/
private @Nullable RedshiftUnloadSettingsResponse redshiftUnloadSettings;
/**
* @return Source retry count. Type: integer (or Expression with resultType integer).
*
*/
private @Nullable Object sourceRetryCount;
/**
* @return Source retry wait. Type: string (or Expression with resultType string), pattern: ((\d+)\.)?(\d\d):(60|([0-5][0-9])):(60|([0-5][0-9])).
*
*/
private @Nullable Object sourceRetryWait;
/**
* @return Copy source type.
* Expected value is 'AmazonRedshiftSource'.
*
*/
private String type;
private AmazonRedshiftSourceResponse() {}
/**
* @return Specifies the additional columns to be added to source data. Type: array of objects(AdditionalColumns) (or Expression with resultType array of objects).
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy