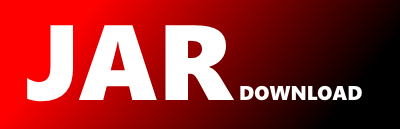
com.pulumi.azurenative.datafactory.outputs.ValidationActivityResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datafactory.outputs;
import com.pulumi.azurenative.datafactory.outputs.ActivityDependencyResponse;
import com.pulumi.azurenative.datafactory.outputs.DatasetReferenceResponse;
import com.pulumi.azurenative.datafactory.outputs.UserPropertyResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ValidationActivityResponse {
/**
* @return Can be used if dataset points to a folder. If set to true, the folder must have at least one file. If set to false, the folder must be empty. Type: boolean (or Expression with resultType boolean).
*
*/
private @Nullable Object childItems;
/**
* @return Validation activity dataset reference.
*
*/
private DatasetReferenceResponse dataset;
/**
* @return Activity depends on condition.
*
*/
private @Nullable List dependsOn;
/**
* @return Activity description.
*
*/
private @Nullable String description;
/**
* @return Can be used if dataset points to a file. The file must be greater than or equal in size to the value specified. Type: integer (or Expression with resultType integer).
*
*/
private @Nullable Object minimumSize;
/**
* @return Activity name.
*
*/
private String name;
/**
* @return Status result of the activity when the state is set to Inactive. This is an optional property and if not provided when the activity is inactive, the status will be Succeeded by default.
*
*/
private @Nullable String onInactiveMarkAs;
/**
* @return A delay in seconds between validation attempts. If no value is specified, 10 seconds will be used as the default. Type: integer (or Expression with resultType integer).
*
*/
private @Nullable Object sleep;
/**
* @return Activity state. This is an optional property and if not provided, the state will be Active by default.
*
*/
private @Nullable String state;
/**
* @return Specifies the timeout for the activity to run. If there is no value specified, it takes the value of TimeSpan.FromDays(7) which is 1 week as default. Type: string (or Expression with resultType string), pattern: ((\d+)\.)?(\d\d):(60|([0-5][0-9])):(60|([0-5][0-9])).
*
*/
private @Nullable Object timeout;
/**
* @return Type of activity.
* Expected value is 'Validation'.
*
*/
private String type;
/**
* @return Activity user properties.
*
*/
private @Nullable List userProperties;
private ValidationActivityResponse() {}
/**
* @return Can be used if dataset points to a folder. If set to true, the folder must have at least one file. If set to false, the folder must be empty. Type: boolean (or Expression with resultType boolean).
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy