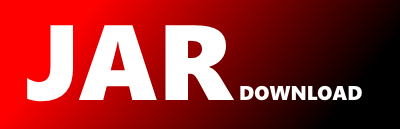
com.pulumi.azurenative.datamigration.outputs.MigrateSqlServerSqlDbSyncTaskOutputMigrationLevelResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datamigration.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class MigrateSqlServerSqlDbSyncTaskOutputMigrationLevelResponse {
/**
* @return Count of databases
*
*/
private Integer databaseCount;
/**
* @return Migration end time
*
*/
private String endedOn;
/**
* @return Result identifier
*
*/
private String id;
/**
* @return Result type
* Expected value is 'MigrationLevelOutput'.
*
*/
private String resultType;
/**
* @return Source server name
*
*/
private String sourceServer;
/**
* @return Source server version
*
*/
private String sourceServerVersion;
/**
* @return Migration start time
*
*/
private String startedOn;
/**
* @return Target server name
*
*/
private String targetServer;
/**
* @return Target server version
*
*/
private String targetServerVersion;
private MigrateSqlServerSqlDbSyncTaskOutputMigrationLevelResponse() {}
/**
* @return Count of databases
*
*/
public Integer databaseCount() {
return this.databaseCount;
}
/**
* @return Migration end time
*
*/
public String endedOn() {
return this.endedOn;
}
/**
* @return Result identifier
*
*/
public String id() {
return this.id;
}
/**
* @return Result type
* Expected value is 'MigrationLevelOutput'.
*
*/
public String resultType() {
return this.resultType;
}
/**
* @return Source server name
*
*/
public String sourceServer() {
return this.sourceServer;
}
/**
* @return Source server version
*
*/
public String sourceServerVersion() {
return this.sourceServerVersion;
}
/**
* @return Migration start time
*
*/
public String startedOn() {
return this.startedOn;
}
/**
* @return Target server name
*
*/
public String targetServer() {
return this.targetServer;
}
/**
* @return Target server version
*
*/
public String targetServerVersion() {
return this.targetServerVersion;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(MigrateSqlServerSqlDbSyncTaskOutputMigrationLevelResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Integer databaseCount;
private String endedOn;
private String id;
private String resultType;
private String sourceServer;
private String sourceServerVersion;
private String startedOn;
private String targetServer;
private String targetServerVersion;
public Builder() {}
public Builder(MigrateSqlServerSqlDbSyncTaskOutputMigrationLevelResponse defaults) {
Objects.requireNonNull(defaults);
this.databaseCount = defaults.databaseCount;
this.endedOn = defaults.endedOn;
this.id = defaults.id;
this.resultType = defaults.resultType;
this.sourceServer = defaults.sourceServer;
this.sourceServerVersion = defaults.sourceServerVersion;
this.startedOn = defaults.startedOn;
this.targetServer = defaults.targetServer;
this.targetServerVersion = defaults.targetServerVersion;
}
@CustomType.Setter
public Builder databaseCount(Integer databaseCount) {
if (databaseCount == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbSyncTaskOutputMigrationLevelResponse", "databaseCount");
}
this.databaseCount = databaseCount;
return this;
}
@CustomType.Setter
public Builder endedOn(String endedOn) {
if (endedOn == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbSyncTaskOutputMigrationLevelResponse", "endedOn");
}
this.endedOn = endedOn;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbSyncTaskOutputMigrationLevelResponse", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder resultType(String resultType) {
if (resultType == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbSyncTaskOutputMigrationLevelResponse", "resultType");
}
this.resultType = resultType;
return this;
}
@CustomType.Setter
public Builder sourceServer(String sourceServer) {
if (sourceServer == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbSyncTaskOutputMigrationLevelResponse", "sourceServer");
}
this.sourceServer = sourceServer;
return this;
}
@CustomType.Setter
public Builder sourceServerVersion(String sourceServerVersion) {
if (sourceServerVersion == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbSyncTaskOutputMigrationLevelResponse", "sourceServerVersion");
}
this.sourceServerVersion = sourceServerVersion;
return this;
}
@CustomType.Setter
public Builder startedOn(String startedOn) {
if (startedOn == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbSyncTaskOutputMigrationLevelResponse", "startedOn");
}
this.startedOn = startedOn;
return this;
}
@CustomType.Setter
public Builder targetServer(String targetServer) {
if (targetServer == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbSyncTaskOutputMigrationLevelResponse", "targetServer");
}
this.targetServer = targetServer;
return this;
}
@CustomType.Setter
public Builder targetServerVersion(String targetServerVersion) {
if (targetServerVersion == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbSyncTaskOutputMigrationLevelResponse", "targetServerVersion");
}
this.targetServerVersion = targetServerVersion;
return this;
}
public MigrateSqlServerSqlDbSyncTaskOutputMigrationLevelResponse build() {
final var _resultValue = new MigrateSqlServerSqlDbSyncTaskOutputMigrationLevelResponse();
_resultValue.databaseCount = databaseCount;
_resultValue.endedOn = endedOn;
_resultValue.id = id;
_resultValue.resultType = resultType;
_resultValue.sourceServer = sourceServer;
_resultValue.sourceServerVersion = sourceServerVersion;
_resultValue.startedOn = startedOn;
_resultValue.targetServer = targetServer;
_resultValue.targetServerVersion = targetServerVersion;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy