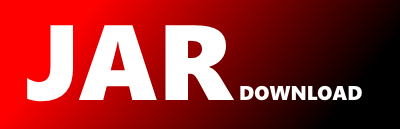
com.pulumi.azurenative.datamigration.outputs.MongoDbMigrationProgressResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datamigration.outputs;
import com.pulumi.azurenative.datamigration.outputs.MongoDbDatabaseProgressResponse;
import com.pulumi.azurenative.datamigration.outputs.MongoDbErrorResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class MongoDbMigrationProgressResponse {
/**
* @return The number of document bytes copied during the Copying stage
*
*/
private Double bytesCopied;
/**
* @return The progress of the databases in the migration. The keys are the names of the databases
*
*/
private @Nullable Map databases;
/**
* @return The number of documents copied during the Copying stage
*
*/
private Double documentsCopied;
/**
* @return The elapsed time in the format [ddd.]hh:mm:ss[.fffffff] (i.e. TimeSpan format)
*
*/
private String elapsedTime;
/**
* @return The errors and warnings that have occurred for the current object. The keys are the error codes.
*
*/
private Map errors;
/**
* @return The number of oplog events awaiting replay
*
*/
private Double eventsPending;
/**
* @return The number of oplog events replayed so far
*
*/
private Double eventsReplayed;
/**
* @return The timestamp of the last oplog event received, or null if no oplog event has been received yet
*
*/
private @Nullable String lastEventTime;
/**
* @return The timestamp of the last oplog event replayed, or null if no oplog event has been replayed yet
*
*/
private @Nullable String lastReplayTime;
/**
* @return The name of the progress object. For a collection, this is the unqualified collection name. For a database, this is the database name. For the overall migration, this is null.
*
*/
private @Nullable String name;
/**
* @return The qualified name of the progress object. For a collection, this is the database-qualified name. For a database, this is the database name. For the overall migration, this is null.
*
*/
private @Nullable String qualifiedName;
/**
* @return The type of progress object
* Expected value is 'Migration'.
*
*/
private String resultType;
private String state;
/**
* @return The total number of document bytes on the source at the beginning of the Copying stage, or -1 if the total size was unknown
*
*/
private Double totalBytes;
/**
* @return The total number of documents on the source at the beginning of the Copying stage, or -1 if the total count was unknown
*
*/
private Double totalDocuments;
private MongoDbMigrationProgressResponse() {}
/**
* @return The number of document bytes copied during the Copying stage
*
*/
public Double bytesCopied() {
return this.bytesCopied;
}
/**
* @return The progress of the databases in the migration. The keys are the names of the databases
*
*/
public Map databases() {
return this.databases == null ? Map.of() : this.databases;
}
/**
* @return The number of documents copied during the Copying stage
*
*/
public Double documentsCopied() {
return this.documentsCopied;
}
/**
* @return The elapsed time in the format [ddd.]hh:mm:ss[.fffffff] (i.e. TimeSpan format)
*
*/
public String elapsedTime() {
return this.elapsedTime;
}
/**
* @return The errors and warnings that have occurred for the current object. The keys are the error codes.
*
*/
public Map errors() {
return this.errors;
}
/**
* @return The number of oplog events awaiting replay
*
*/
public Double eventsPending() {
return this.eventsPending;
}
/**
* @return The number of oplog events replayed so far
*
*/
public Double eventsReplayed() {
return this.eventsReplayed;
}
/**
* @return The timestamp of the last oplog event received, or null if no oplog event has been received yet
*
*/
public Optional lastEventTime() {
return Optional.ofNullable(this.lastEventTime);
}
/**
* @return The timestamp of the last oplog event replayed, or null if no oplog event has been replayed yet
*
*/
public Optional lastReplayTime() {
return Optional.ofNullable(this.lastReplayTime);
}
/**
* @return The name of the progress object. For a collection, this is the unqualified collection name. For a database, this is the database name. For the overall migration, this is null.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return The qualified name of the progress object. For a collection, this is the database-qualified name. For a database, this is the database name. For the overall migration, this is null.
*
*/
public Optional qualifiedName() {
return Optional.ofNullable(this.qualifiedName);
}
/**
* @return The type of progress object
* Expected value is 'Migration'.
*
*/
public String resultType() {
return this.resultType;
}
public String state() {
return this.state;
}
/**
* @return The total number of document bytes on the source at the beginning of the Copying stage, or -1 if the total size was unknown
*
*/
public Double totalBytes() {
return this.totalBytes;
}
/**
* @return The total number of documents on the source at the beginning of the Copying stage, or -1 if the total count was unknown
*
*/
public Double totalDocuments() {
return this.totalDocuments;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(MongoDbMigrationProgressResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Double bytesCopied;
private @Nullable Map databases;
private Double documentsCopied;
private String elapsedTime;
private Map errors;
private Double eventsPending;
private Double eventsReplayed;
private @Nullable String lastEventTime;
private @Nullable String lastReplayTime;
private @Nullable String name;
private @Nullable String qualifiedName;
private String resultType;
private String state;
private Double totalBytes;
private Double totalDocuments;
public Builder() {}
public Builder(MongoDbMigrationProgressResponse defaults) {
Objects.requireNonNull(defaults);
this.bytesCopied = defaults.bytesCopied;
this.databases = defaults.databases;
this.documentsCopied = defaults.documentsCopied;
this.elapsedTime = defaults.elapsedTime;
this.errors = defaults.errors;
this.eventsPending = defaults.eventsPending;
this.eventsReplayed = defaults.eventsReplayed;
this.lastEventTime = defaults.lastEventTime;
this.lastReplayTime = defaults.lastReplayTime;
this.name = defaults.name;
this.qualifiedName = defaults.qualifiedName;
this.resultType = defaults.resultType;
this.state = defaults.state;
this.totalBytes = defaults.totalBytes;
this.totalDocuments = defaults.totalDocuments;
}
@CustomType.Setter
public Builder bytesCopied(Double bytesCopied) {
if (bytesCopied == null) {
throw new MissingRequiredPropertyException("MongoDbMigrationProgressResponse", "bytesCopied");
}
this.bytesCopied = bytesCopied;
return this;
}
@CustomType.Setter
public Builder databases(@Nullable Map databases) {
this.databases = databases;
return this;
}
@CustomType.Setter
public Builder documentsCopied(Double documentsCopied) {
if (documentsCopied == null) {
throw new MissingRequiredPropertyException("MongoDbMigrationProgressResponse", "documentsCopied");
}
this.documentsCopied = documentsCopied;
return this;
}
@CustomType.Setter
public Builder elapsedTime(String elapsedTime) {
if (elapsedTime == null) {
throw new MissingRequiredPropertyException("MongoDbMigrationProgressResponse", "elapsedTime");
}
this.elapsedTime = elapsedTime;
return this;
}
@CustomType.Setter
public Builder errors(Map errors) {
if (errors == null) {
throw new MissingRequiredPropertyException("MongoDbMigrationProgressResponse", "errors");
}
this.errors = errors;
return this;
}
@CustomType.Setter
public Builder eventsPending(Double eventsPending) {
if (eventsPending == null) {
throw new MissingRequiredPropertyException("MongoDbMigrationProgressResponse", "eventsPending");
}
this.eventsPending = eventsPending;
return this;
}
@CustomType.Setter
public Builder eventsReplayed(Double eventsReplayed) {
if (eventsReplayed == null) {
throw new MissingRequiredPropertyException("MongoDbMigrationProgressResponse", "eventsReplayed");
}
this.eventsReplayed = eventsReplayed;
return this;
}
@CustomType.Setter
public Builder lastEventTime(@Nullable String lastEventTime) {
this.lastEventTime = lastEventTime;
return this;
}
@CustomType.Setter
public Builder lastReplayTime(@Nullable String lastReplayTime) {
this.lastReplayTime = lastReplayTime;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder qualifiedName(@Nullable String qualifiedName) {
this.qualifiedName = qualifiedName;
return this;
}
@CustomType.Setter
public Builder resultType(String resultType) {
if (resultType == null) {
throw new MissingRequiredPropertyException("MongoDbMigrationProgressResponse", "resultType");
}
this.resultType = resultType;
return this;
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("MongoDbMigrationProgressResponse", "state");
}
this.state = state;
return this;
}
@CustomType.Setter
public Builder totalBytes(Double totalBytes) {
if (totalBytes == null) {
throw new MissingRequiredPropertyException("MongoDbMigrationProgressResponse", "totalBytes");
}
this.totalBytes = totalBytes;
return this;
}
@CustomType.Setter
public Builder totalDocuments(Double totalDocuments) {
if (totalDocuments == null) {
throw new MissingRequiredPropertyException("MongoDbMigrationProgressResponse", "totalDocuments");
}
this.totalDocuments = totalDocuments;
return this;
}
public MongoDbMigrationProgressResponse build() {
final var _resultValue = new MongoDbMigrationProgressResponse();
_resultValue.bytesCopied = bytesCopied;
_resultValue.databases = databases;
_resultValue.documentsCopied = documentsCopied;
_resultValue.elapsedTime = elapsedTime;
_resultValue.errors = errors;
_resultValue.eventsPending = eventsPending;
_resultValue.eventsReplayed = eventsReplayed;
_resultValue.lastEventTime = lastEventTime;
_resultValue.lastReplayTime = lastReplayTime;
_resultValue.name = name;
_resultValue.qualifiedName = qualifiedName;
_resultValue.resultType = resultType;
_resultValue.state = state;
_resultValue.totalBytes = totalBytes;
_resultValue.totalDocuments = totalDocuments;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy