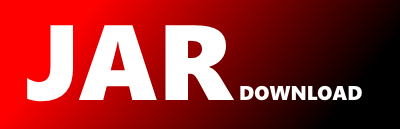
com.pulumi.azurenative.datamigration.outputs.MongoDbMigrationSettingsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datamigration.outputs;
import com.pulumi.azurenative.datamigration.outputs.MongoDbConnectionInfoResponse;
import com.pulumi.azurenative.datamigration.outputs.MongoDbDatabaseSettingsResponse;
import com.pulumi.azurenative.datamigration.outputs.MongoDbThrottlingSettingsResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class MongoDbMigrationSettingsResponse {
/**
* @return The RU limit on a CosmosDB target that collections will be temporarily increased to (if lower) during the initial copy of a migration, from 10,000 to 1,000,000, or 0 to use the default boost (which is generally the maximum), or null to not boost the RUs. This setting has no effect on non-CosmosDB targets.
*
*/
private @Nullable Integer boostRUs;
/**
* @return The databases on the source cluster to migrate to the target. The keys are the names of the databases.
*
*/
private Map databases;
/**
* @return Describes how changes will be replicated from the source to the target. The default is OneTime.
*
*/
private @Nullable String replication;
/**
* @return Settings used to connect to the source cluster
*
*/
private MongoDbConnectionInfoResponse source;
/**
* @return Settings used to connect to the target cluster
*
*/
private MongoDbConnectionInfoResponse target;
/**
* @return Settings used to limit the resource usage of the migration
*
*/
private @Nullable MongoDbThrottlingSettingsResponse throttling;
private MongoDbMigrationSettingsResponse() {}
/**
* @return The RU limit on a CosmosDB target that collections will be temporarily increased to (if lower) during the initial copy of a migration, from 10,000 to 1,000,000, or 0 to use the default boost (which is generally the maximum), or null to not boost the RUs. This setting has no effect on non-CosmosDB targets.
*
*/
public Optional boostRUs() {
return Optional.ofNullable(this.boostRUs);
}
/**
* @return The databases on the source cluster to migrate to the target. The keys are the names of the databases.
*
*/
public Map databases() {
return this.databases;
}
/**
* @return Describes how changes will be replicated from the source to the target. The default is OneTime.
*
*/
public Optional replication() {
return Optional.ofNullable(this.replication);
}
/**
* @return Settings used to connect to the source cluster
*
*/
public MongoDbConnectionInfoResponse source() {
return this.source;
}
/**
* @return Settings used to connect to the target cluster
*
*/
public MongoDbConnectionInfoResponse target() {
return this.target;
}
/**
* @return Settings used to limit the resource usage of the migration
*
*/
public Optional throttling() {
return Optional.ofNullable(this.throttling);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(MongoDbMigrationSettingsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Integer boostRUs;
private Map databases;
private @Nullable String replication;
private MongoDbConnectionInfoResponse source;
private MongoDbConnectionInfoResponse target;
private @Nullable MongoDbThrottlingSettingsResponse throttling;
public Builder() {}
public Builder(MongoDbMigrationSettingsResponse defaults) {
Objects.requireNonNull(defaults);
this.boostRUs = defaults.boostRUs;
this.databases = defaults.databases;
this.replication = defaults.replication;
this.source = defaults.source;
this.target = defaults.target;
this.throttling = defaults.throttling;
}
@CustomType.Setter
public Builder boostRUs(@Nullable Integer boostRUs) {
this.boostRUs = boostRUs;
return this;
}
@CustomType.Setter
public Builder databases(Map databases) {
if (databases == null) {
throw new MissingRequiredPropertyException("MongoDbMigrationSettingsResponse", "databases");
}
this.databases = databases;
return this;
}
@CustomType.Setter
public Builder replication(@Nullable String replication) {
this.replication = replication;
return this;
}
@CustomType.Setter
public Builder source(MongoDbConnectionInfoResponse source) {
if (source == null) {
throw new MissingRequiredPropertyException("MongoDbMigrationSettingsResponse", "source");
}
this.source = source;
return this;
}
@CustomType.Setter
public Builder target(MongoDbConnectionInfoResponse target) {
if (target == null) {
throw new MissingRequiredPropertyException("MongoDbMigrationSettingsResponse", "target");
}
this.target = target;
return this;
}
@CustomType.Setter
public Builder throttling(@Nullable MongoDbThrottlingSettingsResponse throttling) {
this.throttling = throttling;
return this;
}
public MongoDbMigrationSettingsResponse build() {
final var _resultValue = new MongoDbMigrationSettingsResponse();
_resultValue.boostRUs = boostRUs;
_resultValue.databases = databases;
_resultValue.replication = replication;
_resultValue.source = source;
_resultValue.target = target;
_resultValue.throttling = throttling;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy