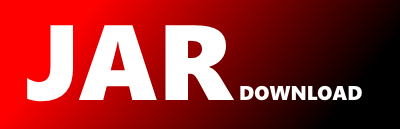
com.pulumi.azurenative.dbforpostgresql.ServerArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.dbforpostgresql;
import com.pulumi.azurenative.dbforpostgresql.enums.CreateMode;
import com.pulumi.azurenative.dbforpostgresql.enums.ReplicationRole;
import com.pulumi.azurenative.dbforpostgresql.enums.ServerVersion;
import com.pulumi.azurenative.dbforpostgresql.inputs.AuthConfigArgs;
import com.pulumi.azurenative.dbforpostgresql.inputs.BackupArgs;
import com.pulumi.azurenative.dbforpostgresql.inputs.DataEncryptionArgs;
import com.pulumi.azurenative.dbforpostgresql.inputs.HighAvailabilityArgs;
import com.pulumi.azurenative.dbforpostgresql.inputs.MaintenanceWindowArgs;
import com.pulumi.azurenative.dbforpostgresql.inputs.NetworkArgs;
import com.pulumi.azurenative.dbforpostgresql.inputs.SkuArgs;
import com.pulumi.azurenative.dbforpostgresql.inputs.StorageArgs;
import com.pulumi.azurenative.dbforpostgresql.inputs.UserAssignedIdentityArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ServerArgs extends com.pulumi.resources.ResourceArgs {
public static final ServerArgs Empty = new ServerArgs();
/**
* The administrator's login name of a server. Can only be specified when the server is being created (and is required for creation).
*
*/
@Import(name="administratorLogin")
private @Nullable Output administratorLogin;
/**
* @return The administrator's login name of a server. Can only be specified when the server is being created (and is required for creation).
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy