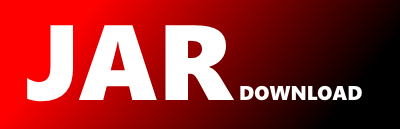
com.pulumi.azurenative.deviceregistry.DeviceregistryFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.deviceregistry;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.deviceregistry.inputs.GetAssetArgs;
import com.pulumi.azurenative.deviceregistry.inputs.GetAssetEndpointProfileArgs;
import com.pulumi.azurenative.deviceregistry.inputs.GetAssetEndpointProfilePlainArgs;
import com.pulumi.azurenative.deviceregistry.inputs.GetAssetPlainArgs;
import com.pulumi.azurenative.deviceregistry.inputs.GetDiscoveredAssetArgs;
import com.pulumi.azurenative.deviceregistry.inputs.GetDiscoveredAssetEndpointProfileArgs;
import com.pulumi.azurenative.deviceregistry.inputs.GetDiscoveredAssetEndpointProfilePlainArgs;
import com.pulumi.azurenative.deviceregistry.inputs.GetDiscoveredAssetPlainArgs;
import com.pulumi.azurenative.deviceregistry.inputs.GetSchemaArgs;
import com.pulumi.azurenative.deviceregistry.inputs.GetSchemaPlainArgs;
import com.pulumi.azurenative.deviceregistry.inputs.GetSchemaRegistryArgs;
import com.pulumi.azurenative.deviceregistry.inputs.GetSchemaRegistryPlainArgs;
import com.pulumi.azurenative.deviceregistry.inputs.GetSchemaVersionArgs;
import com.pulumi.azurenative.deviceregistry.inputs.GetSchemaVersionPlainArgs;
import com.pulumi.azurenative.deviceregistry.outputs.GetAssetEndpointProfileResult;
import com.pulumi.azurenative.deviceregistry.outputs.GetAssetResult;
import com.pulumi.azurenative.deviceregistry.outputs.GetDiscoveredAssetEndpointProfileResult;
import com.pulumi.azurenative.deviceregistry.outputs.GetDiscoveredAssetResult;
import com.pulumi.azurenative.deviceregistry.outputs.GetSchemaRegistryResult;
import com.pulumi.azurenative.deviceregistry.outputs.GetSchemaResult;
import com.pulumi.azurenative.deviceregistry.outputs.GetSchemaVersionResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class DeviceregistryFunctions {
/**
* Get a Asset
* Azure REST API version: 2023-11-01-preview.
*
* Other available API versions: 2024-09-01-preview, 2024-11-01.
*
*/
public static Output getAsset(GetAssetArgs args) {
return getAsset(args, InvokeOptions.Empty);
}
/**
* Get a Asset
* Azure REST API version: 2023-11-01-preview.
*
* Other available API versions: 2024-09-01-preview, 2024-11-01.
*
*/
public static CompletableFuture getAssetPlain(GetAssetPlainArgs args) {
return getAssetPlain(args, InvokeOptions.Empty);
}
/**
* Get a Asset
* Azure REST API version: 2023-11-01-preview.
*
* Other available API versions: 2024-09-01-preview, 2024-11-01.
*
*/
public static Output getAsset(GetAssetArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:deviceregistry:getAsset", TypeShape.of(GetAssetResult.class), args, Utilities.withVersion(options));
}
/**
* Get a Asset
* Azure REST API version: 2023-11-01-preview.
*
* Other available API versions: 2024-09-01-preview, 2024-11-01.
*
*/
public static CompletableFuture getAssetPlain(GetAssetPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:deviceregistry:getAsset", TypeShape.of(GetAssetResult.class), args, Utilities.withVersion(options));
}
/**
* Get a AssetEndpointProfile
* Azure REST API version: 2023-11-01-preview.
*
* Other available API versions: 2024-09-01-preview, 2024-11-01.
*
*/
public static Output getAssetEndpointProfile(GetAssetEndpointProfileArgs args) {
return getAssetEndpointProfile(args, InvokeOptions.Empty);
}
/**
* Get a AssetEndpointProfile
* Azure REST API version: 2023-11-01-preview.
*
* Other available API versions: 2024-09-01-preview, 2024-11-01.
*
*/
public static CompletableFuture getAssetEndpointProfilePlain(GetAssetEndpointProfilePlainArgs args) {
return getAssetEndpointProfilePlain(args, InvokeOptions.Empty);
}
/**
* Get a AssetEndpointProfile
* Azure REST API version: 2023-11-01-preview.
*
* Other available API versions: 2024-09-01-preview, 2024-11-01.
*
*/
public static Output getAssetEndpointProfile(GetAssetEndpointProfileArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:deviceregistry:getAssetEndpointProfile", TypeShape.of(GetAssetEndpointProfileResult.class), args, Utilities.withVersion(options));
}
/**
* Get a AssetEndpointProfile
* Azure REST API version: 2023-11-01-preview.
*
* Other available API versions: 2024-09-01-preview, 2024-11-01.
*
*/
public static CompletableFuture getAssetEndpointProfilePlain(GetAssetEndpointProfilePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:deviceregistry:getAssetEndpointProfile", TypeShape.of(GetAssetEndpointProfileResult.class), args, Utilities.withVersion(options));
}
/**
* Get a DiscoveredAsset
* Azure REST API version: 2024-09-01-preview.
*
*/
public static Output getDiscoveredAsset(GetDiscoveredAssetArgs args) {
return getDiscoveredAsset(args, InvokeOptions.Empty);
}
/**
* Get a DiscoveredAsset
* Azure REST API version: 2024-09-01-preview.
*
*/
public static CompletableFuture getDiscoveredAssetPlain(GetDiscoveredAssetPlainArgs args) {
return getDiscoveredAssetPlain(args, InvokeOptions.Empty);
}
/**
* Get a DiscoveredAsset
* Azure REST API version: 2024-09-01-preview.
*
*/
public static Output getDiscoveredAsset(GetDiscoveredAssetArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:deviceregistry:getDiscoveredAsset", TypeShape.of(GetDiscoveredAssetResult.class), args, Utilities.withVersion(options));
}
/**
* Get a DiscoveredAsset
* Azure REST API version: 2024-09-01-preview.
*
*/
public static CompletableFuture getDiscoveredAssetPlain(GetDiscoveredAssetPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:deviceregistry:getDiscoveredAsset", TypeShape.of(GetDiscoveredAssetResult.class), args, Utilities.withVersion(options));
}
/**
* Get a DiscoveredAssetEndpointProfile
* Azure REST API version: 2024-09-01-preview.
*
*/
public static Output getDiscoveredAssetEndpointProfile(GetDiscoveredAssetEndpointProfileArgs args) {
return getDiscoveredAssetEndpointProfile(args, InvokeOptions.Empty);
}
/**
* Get a DiscoveredAssetEndpointProfile
* Azure REST API version: 2024-09-01-preview.
*
*/
public static CompletableFuture getDiscoveredAssetEndpointProfilePlain(GetDiscoveredAssetEndpointProfilePlainArgs args) {
return getDiscoveredAssetEndpointProfilePlain(args, InvokeOptions.Empty);
}
/**
* Get a DiscoveredAssetEndpointProfile
* Azure REST API version: 2024-09-01-preview.
*
*/
public static Output getDiscoveredAssetEndpointProfile(GetDiscoveredAssetEndpointProfileArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:deviceregistry:getDiscoveredAssetEndpointProfile", TypeShape.of(GetDiscoveredAssetEndpointProfileResult.class), args, Utilities.withVersion(options));
}
/**
* Get a DiscoveredAssetEndpointProfile
* Azure REST API version: 2024-09-01-preview.
*
*/
public static CompletableFuture getDiscoveredAssetEndpointProfilePlain(GetDiscoveredAssetEndpointProfilePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:deviceregistry:getDiscoveredAssetEndpointProfile", TypeShape.of(GetDiscoveredAssetEndpointProfileResult.class), args, Utilities.withVersion(options));
}
/**
* Get a Schema
* Azure REST API version: 2024-09-01-preview.
*
*/
public static Output getSchema(GetSchemaArgs args) {
return getSchema(args, InvokeOptions.Empty);
}
/**
* Get a Schema
* Azure REST API version: 2024-09-01-preview.
*
*/
public static CompletableFuture getSchemaPlain(GetSchemaPlainArgs args) {
return getSchemaPlain(args, InvokeOptions.Empty);
}
/**
* Get a Schema
* Azure REST API version: 2024-09-01-preview.
*
*/
public static Output getSchema(GetSchemaArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:deviceregistry:getSchema", TypeShape.of(GetSchemaResult.class), args, Utilities.withVersion(options));
}
/**
* Get a Schema
* Azure REST API version: 2024-09-01-preview.
*
*/
public static CompletableFuture getSchemaPlain(GetSchemaPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:deviceregistry:getSchema", TypeShape.of(GetSchemaResult.class), args, Utilities.withVersion(options));
}
/**
* Get a SchemaRegistry
* Azure REST API version: 2024-09-01-preview.
*
*/
public static Output getSchemaRegistry(GetSchemaRegistryArgs args) {
return getSchemaRegistry(args, InvokeOptions.Empty);
}
/**
* Get a SchemaRegistry
* Azure REST API version: 2024-09-01-preview.
*
*/
public static CompletableFuture getSchemaRegistryPlain(GetSchemaRegistryPlainArgs args) {
return getSchemaRegistryPlain(args, InvokeOptions.Empty);
}
/**
* Get a SchemaRegistry
* Azure REST API version: 2024-09-01-preview.
*
*/
public static Output getSchemaRegistry(GetSchemaRegistryArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:deviceregistry:getSchemaRegistry", TypeShape.of(GetSchemaRegistryResult.class), args, Utilities.withVersion(options));
}
/**
* Get a SchemaRegistry
* Azure REST API version: 2024-09-01-preview.
*
*/
public static CompletableFuture getSchemaRegistryPlain(GetSchemaRegistryPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:deviceregistry:getSchemaRegistry", TypeShape.of(GetSchemaRegistryResult.class), args, Utilities.withVersion(options));
}
/**
* Get a SchemaVersion
* Azure REST API version: 2024-09-01-preview.
*
*/
public static Output getSchemaVersion(GetSchemaVersionArgs args) {
return getSchemaVersion(args, InvokeOptions.Empty);
}
/**
* Get a SchemaVersion
* Azure REST API version: 2024-09-01-preview.
*
*/
public static CompletableFuture getSchemaVersionPlain(GetSchemaVersionPlainArgs args) {
return getSchemaVersionPlain(args, InvokeOptions.Empty);
}
/**
* Get a SchemaVersion
* Azure REST API version: 2024-09-01-preview.
*
*/
public static Output getSchemaVersion(GetSchemaVersionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:deviceregistry:getSchemaVersion", TypeShape.of(GetSchemaVersionResult.class), args, Utilities.withVersion(options));
}
/**
* Get a SchemaVersion
* Azure REST API version: 2024-09-01-preview.
*
*/
public static CompletableFuture getSchemaVersionPlain(GetSchemaVersionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:deviceregistry:getSchemaVersion", TypeShape.of(GetSchemaVersionResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy