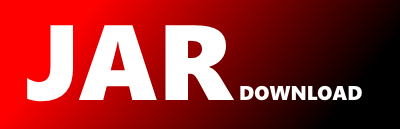
com.pulumi.azurenative.deviceregistry.DiscoveredAssetArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.deviceregistry;
import com.pulumi.azurenative.deviceregistry.inputs.DiscoveredDatasetArgs;
import com.pulumi.azurenative.deviceregistry.inputs.DiscoveredEventArgs;
import com.pulumi.azurenative.deviceregistry.inputs.ExtendedLocationArgs;
import com.pulumi.azurenative.deviceregistry.inputs.TopicArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class DiscoveredAssetArgs extends com.pulumi.resources.ResourceArgs {
public static final DiscoveredAssetArgs Empty = new DiscoveredAssetArgs();
/**
* A reference to the asset endpoint profile (connection information) used by brokers to connect to an endpoint that provides data points for this asset. Must provide asset endpoint profile name.
*
*/
@Import(name="assetEndpointProfileRef", required=true)
private Output assetEndpointProfileRef;
/**
* @return A reference to the asset endpoint profile (connection information) used by brokers to connect to an endpoint that provides data points for this asset. Must provide asset endpoint profile name.
*
*/
public Output assetEndpointProfileRef() {
return this.assetEndpointProfileRef;
}
/**
* Array of datasets that are part of the asset. Each dataset spec describes the data points that make up the set.
*
*/
@Import(name="datasets")
private @Nullable Output> datasets;
/**
* @return Array of datasets that are part of the asset. Each dataset spec describes the data points that make up the set.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy