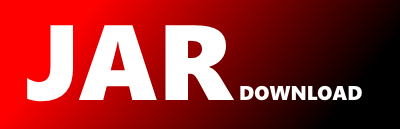
com.pulumi.azurenative.devtestlab.LabArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.devtestlab;
import com.pulumi.azurenative.devtestlab.enums.EnvironmentPermission;
import com.pulumi.azurenative.devtestlab.enums.PremiumDataDisk;
import com.pulumi.azurenative.devtestlab.enums.StorageType;
import com.pulumi.azurenative.devtestlab.inputs.LabAnnouncementPropertiesArgs;
import com.pulumi.azurenative.devtestlab.inputs.LabSupportPropertiesArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class LabArgs extends com.pulumi.resources.ResourceArgs {
public static final LabArgs Empty = new LabArgs();
/**
* The properties of any lab announcement associated with this lab
*
*/
@Import(name="announcement")
private @Nullable Output announcement;
/**
* @return The properties of any lab announcement associated with this lab
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy