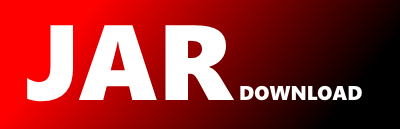
com.pulumi.azurenative.digitaltwins.inputs.AzureDataExplorerConnectionPropertiesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.digitaltwins.inputs;
import com.pulumi.azurenative.digitaltwins.enums.RecordPropertyAndItemRemovals;
import com.pulumi.azurenative.digitaltwins.inputs.ManagedIdentityReferenceArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Properties of a time series database connection to Azure Data Explorer with data being sent via an EventHub.
*
*/
public final class AzureDataExplorerConnectionPropertiesArgs extends com.pulumi.resources.ResourceArgs {
public static final AzureDataExplorerConnectionPropertiesArgs Empty = new AzureDataExplorerConnectionPropertiesArgs();
/**
* The name of the Azure Data Explorer database.
*
*/
@Import(name="adxDatabaseName", required=true)
private Output adxDatabaseName;
/**
* @return The name of the Azure Data Explorer database.
*
*/
public Output adxDatabaseName() {
return this.adxDatabaseName;
}
/**
* The URI of the Azure Data Explorer endpoint.
*
*/
@Import(name="adxEndpointUri", required=true)
private Output adxEndpointUri;
/**
* @return The URI of the Azure Data Explorer endpoint.
*
*/
public Output adxEndpointUri() {
return this.adxEndpointUri;
}
/**
* The name of the Azure Data Explorer table used for recording relationship lifecycle events. The table will not be created if this property is left unspecified.
*
*/
@Import(name="adxRelationshipLifecycleEventsTableName")
private @Nullable Output adxRelationshipLifecycleEventsTableName;
/**
* @return The name of the Azure Data Explorer table used for recording relationship lifecycle events. The table will not be created if this property is left unspecified.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy