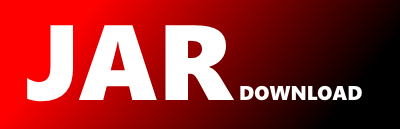
com.pulumi.azurenative.documentdb.inputs.IndexingPolicyArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.documentdb.inputs;
import com.pulumi.azurenative.documentdb.enums.IndexingMode;
import com.pulumi.azurenative.documentdb.inputs.CompositePathArgs;
import com.pulumi.azurenative.documentdb.inputs.ExcludedPathArgs;
import com.pulumi.azurenative.documentdb.inputs.IncludedPathArgs;
import com.pulumi.azurenative.documentdb.inputs.SpatialSpecArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Cosmos DB indexing policy
*
*/
public final class IndexingPolicyArgs extends com.pulumi.resources.ResourceArgs {
public static final IndexingPolicyArgs Empty = new IndexingPolicyArgs();
/**
* Indicates if the indexing policy is automatic
*
*/
@Import(name="automatic")
private @Nullable Output automatic;
/**
* @return Indicates if the indexing policy is automatic
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy