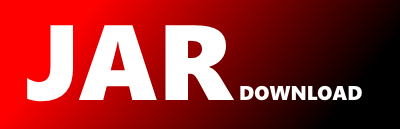
com.pulumi.azurenative.documentdb.outputs.ClusterResourceResponseProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.documentdb.outputs;
import com.pulumi.azurenative.documentdb.outputs.CassandraErrorResponse;
import com.pulumi.azurenative.documentdb.outputs.CertificateResponse;
import com.pulumi.azurenative.documentdb.outputs.SeedNodeResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ClusterResourceResponseProperties {
/**
* @return Which authentication method Cassandra should use to authenticate clients. 'None' turns off authentication, so should not be used except in emergencies. 'Cassandra' is the default password based authentication. The default is 'Cassandra'.
*
*/
private @Nullable String authenticationMethod;
/**
* @return Whether Cassandra audit logging is enabled
*
*/
private @Nullable Boolean cassandraAuditLoggingEnabled;
/**
* @return Which version of Cassandra should this cluster converge to running (e.g., 3.11). When updated, the cluster may take some time to migrate to the new version.
*
*/
private @Nullable String cassandraVersion;
/**
* @return List of TLS certificates used to authorize clients connecting to the cluster. All connections are TLS encrypted whether clientCertificates is set or not, but if clientCertificates is set, the managed Cassandra cluster will reject all connections not bearing a TLS client certificate that can be validated from one or more of the public certificates in this property.
*
*/
private @Nullable List clientCertificates;
/**
* @return If you need to set the clusterName property in cassandra.yaml to something besides the resource name of the cluster, set the value to use on this property.
*
*/
private @Nullable String clusterNameOverride;
/**
* @return Whether the cluster and associated data centers has been deallocated.
*
*/
private @Nullable Boolean deallocated;
/**
* @return Resource id of a subnet that this cluster's management service should have its network interface attached to. The subnet must be routable to all subnets that will be delegated to data centers. The resource id must be of the form '/subscriptions/<subscription id>/resourceGroups/<resource group>/providers/Microsoft.Network/virtualNetworks/<virtual network>/subnets/<subnet>'
*
*/
private @Nullable String delegatedManagementSubnetId;
/**
* @return List of TLS certificates used to authorize gossip from unmanaged data centers. The TLS certificates of all nodes in unmanaged data centers must be verifiable using one of the certificates provided in this property.
*
*/
private @Nullable List externalGossipCertificates;
/**
* @return List of IP addresses of seed nodes in unmanaged data centers. These will be added to the seed node lists of all managed nodes.
*
*/
private @Nullable List externalSeedNodes;
/**
* @return List of TLS certificates that unmanaged nodes must trust for gossip with managed nodes. All managed nodes will present TLS client certificates that are verifiable using one of the certificates provided in this property.
*
*/
private List gossipCertificates;
/**
* @return (Deprecated) Number of hours to wait between taking a backup of the cluster.
*
*/
private @Nullable Integer hoursBetweenBackups;
/**
* @return Hostname or IP address where the Prometheus endpoint containing data about the managed Cassandra nodes can be reached.
*
*/
private @Nullable SeedNodeResponse prometheusEndpoint;
/**
* @return Error related to resource provisioning.
*
*/
private @Nullable CassandraErrorResponse provisionError;
/**
* @return The status of the resource at the time the operation was called.
*
*/
private @Nullable String provisioningState;
/**
* @return Should automatic repairs run on this cluster? If omitted, this is true, and should stay true unless you are running a hybrid cluster where you are already doing your own repairs.
*
*/
private @Nullable Boolean repairEnabled;
/**
* @return List of IP addresses of seed nodes in the managed data centers. These should be added to the seed node lists of all unmanaged nodes.
*
*/
private List seedNodes;
private ClusterResourceResponseProperties() {}
/**
* @return Which authentication method Cassandra should use to authenticate clients. 'None' turns off authentication, so should not be used except in emergencies. 'Cassandra' is the default password based authentication. The default is 'Cassandra'.
*
*/
public Optional authenticationMethod() {
return Optional.ofNullable(this.authenticationMethod);
}
/**
* @return Whether Cassandra audit logging is enabled
*
*/
public Optional cassandraAuditLoggingEnabled() {
return Optional.ofNullable(this.cassandraAuditLoggingEnabled);
}
/**
* @return Which version of Cassandra should this cluster converge to running (e.g., 3.11). When updated, the cluster may take some time to migrate to the new version.
*
*/
public Optional cassandraVersion() {
return Optional.ofNullable(this.cassandraVersion);
}
/**
* @return List of TLS certificates used to authorize clients connecting to the cluster. All connections are TLS encrypted whether clientCertificates is set or not, but if clientCertificates is set, the managed Cassandra cluster will reject all connections not bearing a TLS client certificate that can be validated from one or more of the public certificates in this property.
*
*/
public List clientCertificates() {
return this.clientCertificates == null ? List.of() : this.clientCertificates;
}
/**
* @return If you need to set the clusterName property in cassandra.yaml to something besides the resource name of the cluster, set the value to use on this property.
*
*/
public Optional clusterNameOverride() {
return Optional.ofNullable(this.clusterNameOverride);
}
/**
* @return Whether the cluster and associated data centers has been deallocated.
*
*/
public Optional deallocated() {
return Optional.ofNullable(this.deallocated);
}
/**
* @return Resource id of a subnet that this cluster's management service should have its network interface attached to. The subnet must be routable to all subnets that will be delegated to data centers. The resource id must be of the form '/subscriptions/<subscription id>/resourceGroups/<resource group>/providers/Microsoft.Network/virtualNetworks/<virtual network>/subnets/<subnet>'
*
*/
public Optional delegatedManagementSubnetId() {
return Optional.ofNullable(this.delegatedManagementSubnetId);
}
/**
* @return List of TLS certificates used to authorize gossip from unmanaged data centers. The TLS certificates of all nodes in unmanaged data centers must be verifiable using one of the certificates provided in this property.
*
*/
public List externalGossipCertificates() {
return this.externalGossipCertificates == null ? List.of() : this.externalGossipCertificates;
}
/**
* @return List of IP addresses of seed nodes in unmanaged data centers. These will be added to the seed node lists of all managed nodes.
*
*/
public List externalSeedNodes() {
return this.externalSeedNodes == null ? List.of() : this.externalSeedNodes;
}
/**
* @return List of TLS certificates that unmanaged nodes must trust for gossip with managed nodes. All managed nodes will present TLS client certificates that are verifiable using one of the certificates provided in this property.
*
*/
public List gossipCertificates() {
return this.gossipCertificates;
}
/**
* @return (Deprecated) Number of hours to wait between taking a backup of the cluster.
*
*/
public Optional hoursBetweenBackups() {
return Optional.ofNullable(this.hoursBetweenBackups);
}
/**
* @return Hostname or IP address where the Prometheus endpoint containing data about the managed Cassandra nodes can be reached.
*
*/
public Optional prometheusEndpoint() {
return Optional.ofNullable(this.prometheusEndpoint);
}
/**
* @return Error related to resource provisioning.
*
*/
public Optional provisionError() {
return Optional.ofNullable(this.provisionError);
}
/**
* @return The status of the resource at the time the operation was called.
*
*/
public Optional provisioningState() {
return Optional.ofNullable(this.provisioningState);
}
/**
* @return Should automatic repairs run on this cluster? If omitted, this is true, and should stay true unless you are running a hybrid cluster where you are already doing your own repairs.
*
*/
public Optional repairEnabled() {
return Optional.ofNullable(this.repairEnabled);
}
/**
* @return List of IP addresses of seed nodes in the managed data centers. These should be added to the seed node lists of all unmanaged nodes.
*
*/
public List seedNodes() {
return this.seedNodes;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ClusterResourceResponseProperties defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String authenticationMethod;
private @Nullable Boolean cassandraAuditLoggingEnabled;
private @Nullable String cassandraVersion;
private @Nullable List clientCertificates;
private @Nullable String clusterNameOverride;
private @Nullable Boolean deallocated;
private @Nullable String delegatedManagementSubnetId;
private @Nullable List externalGossipCertificates;
private @Nullable List externalSeedNodes;
private List gossipCertificates;
private @Nullable Integer hoursBetweenBackups;
private @Nullable SeedNodeResponse prometheusEndpoint;
private @Nullable CassandraErrorResponse provisionError;
private @Nullable String provisioningState;
private @Nullable Boolean repairEnabled;
private List seedNodes;
public Builder() {}
public Builder(ClusterResourceResponseProperties defaults) {
Objects.requireNonNull(defaults);
this.authenticationMethod = defaults.authenticationMethod;
this.cassandraAuditLoggingEnabled = defaults.cassandraAuditLoggingEnabled;
this.cassandraVersion = defaults.cassandraVersion;
this.clientCertificates = defaults.clientCertificates;
this.clusterNameOverride = defaults.clusterNameOverride;
this.deallocated = defaults.deallocated;
this.delegatedManagementSubnetId = defaults.delegatedManagementSubnetId;
this.externalGossipCertificates = defaults.externalGossipCertificates;
this.externalSeedNodes = defaults.externalSeedNodes;
this.gossipCertificates = defaults.gossipCertificates;
this.hoursBetweenBackups = defaults.hoursBetweenBackups;
this.prometheusEndpoint = defaults.prometheusEndpoint;
this.provisionError = defaults.provisionError;
this.provisioningState = defaults.provisioningState;
this.repairEnabled = defaults.repairEnabled;
this.seedNodes = defaults.seedNodes;
}
@CustomType.Setter
public Builder authenticationMethod(@Nullable String authenticationMethod) {
this.authenticationMethod = authenticationMethod;
return this;
}
@CustomType.Setter
public Builder cassandraAuditLoggingEnabled(@Nullable Boolean cassandraAuditLoggingEnabled) {
this.cassandraAuditLoggingEnabled = cassandraAuditLoggingEnabled;
return this;
}
@CustomType.Setter
public Builder cassandraVersion(@Nullable String cassandraVersion) {
this.cassandraVersion = cassandraVersion;
return this;
}
@CustomType.Setter
public Builder clientCertificates(@Nullable List clientCertificates) {
this.clientCertificates = clientCertificates;
return this;
}
public Builder clientCertificates(CertificateResponse... clientCertificates) {
return clientCertificates(List.of(clientCertificates));
}
@CustomType.Setter
public Builder clusterNameOverride(@Nullable String clusterNameOverride) {
this.clusterNameOverride = clusterNameOverride;
return this;
}
@CustomType.Setter
public Builder deallocated(@Nullable Boolean deallocated) {
this.deallocated = deallocated;
return this;
}
@CustomType.Setter
public Builder delegatedManagementSubnetId(@Nullable String delegatedManagementSubnetId) {
this.delegatedManagementSubnetId = delegatedManagementSubnetId;
return this;
}
@CustomType.Setter
public Builder externalGossipCertificates(@Nullable List externalGossipCertificates) {
this.externalGossipCertificates = externalGossipCertificates;
return this;
}
public Builder externalGossipCertificates(CertificateResponse... externalGossipCertificates) {
return externalGossipCertificates(List.of(externalGossipCertificates));
}
@CustomType.Setter
public Builder externalSeedNodes(@Nullable List externalSeedNodes) {
this.externalSeedNodes = externalSeedNodes;
return this;
}
public Builder externalSeedNodes(SeedNodeResponse... externalSeedNodes) {
return externalSeedNodes(List.of(externalSeedNodes));
}
@CustomType.Setter
public Builder gossipCertificates(List gossipCertificates) {
if (gossipCertificates == null) {
throw new MissingRequiredPropertyException("ClusterResourceResponseProperties", "gossipCertificates");
}
this.gossipCertificates = gossipCertificates;
return this;
}
public Builder gossipCertificates(CertificateResponse... gossipCertificates) {
return gossipCertificates(List.of(gossipCertificates));
}
@CustomType.Setter
public Builder hoursBetweenBackups(@Nullable Integer hoursBetweenBackups) {
this.hoursBetweenBackups = hoursBetweenBackups;
return this;
}
@CustomType.Setter
public Builder prometheusEndpoint(@Nullable SeedNodeResponse prometheusEndpoint) {
this.prometheusEndpoint = prometheusEndpoint;
return this;
}
@CustomType.Setter
public Builder provisionError(@Nullable CassandraErrorResponse provisionError) {
this.provisionError = provisionError;
return this;
}
@CustomType.Setter
public Builder provisioningState(@Nullable String provisioningState) {
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder repairEnabled(@Nullable Boolean repairEnabled) {
this.repairEnabled = repairEnabled;
return this;
}
@CustomType.Setter
public Builder seedNodes(List seedNodes) {
if (seedNodes == null) {
throw new MissingRequiredPropertyException("ClusterResourceResponseProperties", "seedNodes");
}
this.seedNodes = seedNodes;
return this;
}
public Builder seedNodes(SeedNodeResponse... seedNodes) {
return seedNodes(List.of(seedNodes));
}
public ClusterResourceResponseProperties build() {
final var _resultValue = new ClusterResourceResponseProperties();
_resultValue.authenticationMethod = authenticationMethod;
_resultValue.cassandraAuditLoggingEnabled = cassandraAuditLoggingEnabled;
_resultValue.cassandraVersion = cassandraVersion;
_resultValue.clientCertificates = clientCertificates;
_resultValue.clusterNameOverride = clusterNameOverride;
_resultValue.deallocated = deallocated;
_resultValue.delegatedManagementSubnetId = delegatedManagementSubnetId;
_resultValue.externalGossipCertificates = externalGossipCertificates;
_resultValue.externalSeedNodes = externalSeedNodes;
_resultValue.gossipCertificates = gossipCertificates;
_resultValue.hoursBetweenBackups = hoursBetweenBackups;
_resultValue.prometheusEndpoint = prometheusEndpoint;
_resultValue.provisionError = provisionError;
_resultValue.provisioningState = provisioningState;
_resultValue.repairEnabled = repairEnabled;
_resultValue.seedNodes = seedNodes;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy