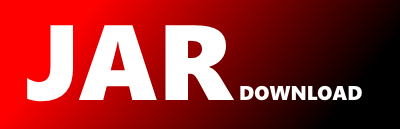
com.pulumi.azurenative.documentdb.outputs.GetMongoDBResourceMongoRoleDefinitionResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.documentdb.outputs;
import com.pulumi.azurenative.documentdb.outputs.PrivilegeResponse;
import com.pulumi.azurenative.documentdb.outputs.RoleResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetMongoDBResourceMongoRoleDefinitionResult {
/**
* @return The database name for which access is being granted for this Role Definition.
*
*/
private @Nullable String databaseName;
/**
* @return The unique resource identifier of the database account.
*
*/
private String id;
/**
* @return The name of the database account.
*
*/
private String name;
/**
* @return A set of privileges contained by the Role Definition. This will allow application of this Role Definition on the entire database account or any underlying Database / Collection. Scopes higher than Database are not enforceable as privilege.
*
*/
private @Nullable List privileges;
/**
* @return A user-friendly name for the Role Definition. Must be unique for the database account.
*
*/
private @Nullable String roleName;
/**
* @return The set of roles inherited by this Role Definition.
*
*/
private @Nullable List roles;
/**
* @return The type of Azure resource.
*
*/
private String type;
private GetMongoDBResourceMongoRoleDefinitionResult() {}
/**
* @return The database name for which access is being granted for this Role Definition.
*
*/
public Optional databaseName() {
return Optional.ofNullable(this.databaseName);
}
/**
* @return The unique resource identifier of the database account.
*
*/
public String id() {
return this.id;
}
/**
* @return The name of the database account.
*
*/
public String name() {
return this.name;
}
/**
* @return A set of privileges contained by the Role Definition. This will allow application of this Role Definition on the entire database account or any underlying Database / Collection. Scopes higher than Database are not enforceable as privilege.
*
*/
public List privileges() {
return this.privileges == null ? List.of() : this.privileges;
}
/**
* @return A user-friendly name for the Role Definition. Must be unique for the database account.
*
*/
public Optional roleName() {
return Optional.ofNullable(this.roleName);
}
/**
* @return The set of roles inherited by this Role Definition.
*
*/
public List roles() {
return this.roles == null ? List.of() : this.roles;
}
/**
* @return The type of Azure resource.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetMongoDBResourceMongoRoleDefinitionResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String databaseName;
private String id;
private String name;
private @Nullable List privileges;
private @Nullable String roleName;
private @Nullable List roles;
private String type;
public Builder() {}
public Builder(GetMongoDBResourceMongoRoleDefinitionResult defaults) {
Objects.requireNonNull(defaults);
this.databaseName = defaults.databaseName;
this.id = defaults.id;
this.name = defaults.name;
this.privileges = defaults.privileges;
this.roleName = defaults.roleName;
this.roles = defaults.roles;
this.type = defaults.type;
}
@CustomType.Setter
public Builder databaseName(@Nullable String databaseName) {
this.databaseName = databaseName;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetMongoDBResourceMongoRoleDefinitionResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetMongoDBResourceMongoRoleDefinitionResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder privileges(@Nullable List privileges) {
this.privileges = privileges;
return this;
}
public Builder privileges(PrivilegeResponse... privileges) {
return privileges(List.of(privileges));
}
@CustomType.Setter
public Builder roleName(@Nullable String roleName) {
this.roleName = roleName;
return this;
}
@CustomType.Setter
public Builder roles(@Nullable List roles) {
this.roles = roles;
return this;
}
public Builder roles(RoleResponse... roles) {
return roles(List.of(roles));
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetMongoDBResourceMongoRoleDefinitionResult", "type");
}
this.type = type;
return this;
}
public GetMongoDBResourceMongoRoleDefinitionResult build() {
final var _resultValue = new GetMongoDBResourceMongoRoleDefinitionResult();
_resultValue.databaseName = databaseName;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.privileges = privileges;
_resultValue.roleName = roleName;
_resultValue.roles = roles;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy