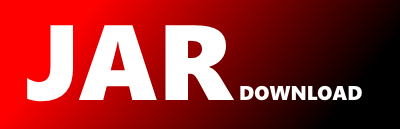
com.pulumi.azurenative.eventhub.outputs.DestinationResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.eventhub.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class DestinationResponse {
/**
* @return Blob naming convention for archive, e.g. {Namespace}/{EventHub}/{PartitionId}/{Year}/{Month}/{Day}/{Hour}/{Minute}/{Second}. Here all the parameters (Namespace,EventHub .. etc) are mandatory irrespective of order
*
*/
private @Nullable String archiveNameFormat;
/**
* @return Blob container Name
*
*/
private @Nullable String blobContainer;
/**
* @return The Azure Data Lake Store name for the captured events
*
*/
private @Nullable String dataLakeAccountName;
/**
* @return The destination folder path for the captured events
*
*/
private @Nullable String dataLakeFolderPath;
/**
* @return Subscription Id of Azure Data Lake Store
*
*/
private @Nullable String dataLakeSubscriptionId;
/**
* @return Name for capture destination
*
*/
private @Nullable String name;
/**
* @return Resource id of the storage account to be used to create the blobs
*
*/
private @Nullable String storageAccountResourceId;
private DestinationResponse() {}
/**
* @return Blob naming convention for archive, e.g. {Namespace}/{EventHub}/{PartitionId}/{Year}/{Month}/{Day}/{Hour}/{Minute}/{Second}. Here all the parameters (Namespace,EventHub .. etc) are mandatory irrespective of order
*
*/
public Optional archiveNameFormat() {
return Optional.ofNullable(this.archiveNameFormat);
}
/**
* @return Blob container Name
*
*/
public Optional blobContainer() {
return Optional.ofNullable(this.blobContainer);
}
/**
* @return The Azure Data Lake Store name for the captured events
*
*/
public Optional dataLakeAccountName() {
return Optional.ofNullable(this.dataLakeAccountName);
}
/**
* @return The destination folder path for the captured events
*
*/
public Optional dataLakeFolderPath() {
return Optional.ofNullable(this.dataLakeFolderPath);
}
/**
* @return Subscription Id of Azure Data Lake Store
*
*/
public Optional dataLakeSubscriptionId() {
return Optional.ofNullable(this.dataLakeSubscriptionId);
}
/**
* @return Name for capture destination
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return Resource id of the storage account to be used to create the blobs
*
*/
public Optional storageAccountResourceId() {
return Optional.ofNullable(this.storageAccountResourceId);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(DestinationResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String archiveNameFormat;
private @Nullable String blobContainer;
private @Nullable String dataLakeAccountName;
private @Nullable String dataLakeFolderPath;
private @Nullable String dataLakeSubscriptionId;
private @Nullable String name;
private @Nullable String storageAccountResourceId;
public Builder() {}
public Builder(DestinationResponse defaults) {
Objects.requireNonNull(defaults);
this.archiveNameFormat = defaults.archiveNameFormat;
this.blobContainer = defaults.blobContainer;
this.dataLakeAccountName = defaults.dataLakeAccountName;
this.dataLakeFolderPath = defaults.dataLakeFolderPath;
this.dataLakeSubscriptionId = defaults.dataLakeSubscriptionId;
this.name = defaults.name;
this.storageAccountResourceId = defaults.storageAccountResourceId;
}
@CustomType.Setter
public Builder archiveNameFormat(@Nullable String archiveNameFormat) {
this.archiveNameFormat = archiveNameFormat;
return this;
}
@CustomType.Setter
public Builder blobContainer(@Nullable String blobContainer) {
this.blobContainer = blobContainer;
return this;
}
@CustomType.Setter
public Builder dataLakeAccountName(@Nullable String dataLakeAccountName) {
this.dataLakeAccountName = dataLakeAccountName;
return this;
}
@CustomType.Setter
public Builder dataLakeFolderPath(@Nullable String dataLakeFolderPath) {
this.dataLakeFolderPath = dataLakeFolderPath;
return this;
}
@CustomType.Setter
public Builder dataLakeSubscriptionId(@Nullable String dataLakeSubscriptionId) {
this.dataLakeSubscriptionId = dataLakeSubscriptionId;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder storageAccountResourceId(@Nullable String storageAccountResourceId) {
this.storageAccountResourceId = storageAccountResourceId;
return this;
}
public DestinationResponse build() {
final var _resultValue = new DestinationResponse();
_resultValue.archiveNameFormat = archiveNameFormat;
_resultValue.blobContainer = blobContainer;
_resultValue.dataLakeAccountName = dataLakeAccountName;
_resultValue.dataLakeFolderPath = dataLakeFolderPath;
_resultValue.dataLakeSubscriptionId = dataLakeSubscriptionId;
_resultValue.name = name;
_resultValue.storageAccountResourceId = storageAccountResourceId;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy