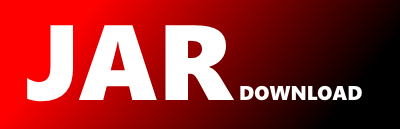
com.pulumi.azurenative.healthcareapis.HealthcareapisFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.healthcareapis;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.healthcareapis.inputs.GetAnalyticsConnectorArgs;
import com.pulumi.azurenative.healthcareapis.inputs.GetAnalyticsConnectorPlainArgs;
import com.pulumi.azurenative.healthcareapis.inputs.GetDicomServiceArgs;
import com.pulumi.azurenative.healthcareapis.inputs.GetDicomServicePlainArgs;
import com.pulumi.azurenative.healthcareapis.inputs.GetFhirServiceArgs;
import com.pulumi.azurenative.healthcareapis.inputs.GetFhirServicePlainArgs;
import com.pulumi.azurenative.healthcareapis.inputs.GetIotConnectorArgs;
import com.pulumi.azurenative.healthcareapis.inputs.GetIotConnectorFhirDestinationArgs;
import com.pulumi.azurenative.healthcareapis.inputs.GetIotConnectorFhirDestinationPlainArgs;
import com.pulumi.azurenative.healthcareapis.inputs.GetIotConnectorPlainArgs;
import com.pulumi.azurenative.healthcareapis.inputs.GetPrivateEndpointConnectionArgs;
import com.pulumi.azurenative.healthcareapis.inputs.GetPrivateEndpointConnectionPlainArgs;
import com.pulumi.azurenative.healthcareapis.inputs.GetServiceArgs;
import com.pulumi.azurenative.healthcareapis.inputs.GetServicePlainArgs;
import com.pulumi.azurenative.healthcareapis.inputs.GetWorkspaceArgs;
import com.pulumi.azurenative.healthcareapis.inputs.GetWorkspacePlainArgs;
import com.pulumi.azurenative.healthcareapis.inputs.GetWorkspacePrivateEndpointConnectionArgs;
import com.pulumi.azurenative.healthcareapis.inputs.GetWorkspacePrivateEndpointConnectionPlainArgs;
import com.pulumi.azurenative.healthcareapis.outputs.GetAnalyticsConnectorResult;
import com.pulumi.azurenative.healthcareapis.outputs.GetDicomServiceResult;
import com.pulumi.azurenative.healthcareapis.outputs.GetFhirServiceResult;
import com.pulumi.azurenative.healthcareapis.outputs.GetIotConnectorFhirDestinationResult;
import com.pulumi.azurenative.healthcareapis.outputs.GetIotConnectorResult;
import com.pulumi.azurenative.healthcareapis.outputs.GetPrivateEndpointConnectionResult;
import com.pulumi.azurenative.healthcareapis.outputs.GetServiceResult;
import com.pulumi.azurenative.healthcareapis.outputs.GetWorkspacePrivateEndpointConnectionResult;
import com.pulumi.azurenative.healthcareapis.outputs.GetWorkspaceResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class HealthcareapisFunctions {
/**
* Gets the properties of the specified Analytics Connector.
* Azure REST API version: 2022-10-01-preview.
*
*/
public static Output getAnalyticsConnector(GetAnalyticsConnectorArgs args) {
return getAnalyticsConnector(args, InvokeOptions.Empty);
}
/**
* Gets the properties of the specified Analytics Connector.
* Azure REST API version: 2022-10-01-preview.
*
*/
public static CompletableFuture getAnalyticsConnectorPlain(GetAnalyticsConnectorPlainArgs args) {
return getAnalyticsConnectorPlain(args, InvokeOptions.Empty);
}
/**
* Gets the properties of the specified Analytics Connector.
* Azure REST API version: 2022-10-01-preview.
*
*/
public static Output getAnalyticsConnector(GetAnalyticsConnectorArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:healthcareapis:getAnalyticsConnector", TypeShape.of(GetAnalyticsConnectorResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of the specified Analytics Connector.
* Azure REST API version: 2022-10-01-preview.
*
*/
public static CompletableFuture getAnalyticsConnectorPlain(GetAnalyticsConnectorPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:healthcareapis:getAnalyticsConnector", TypeShape.of(GetAnalyticsConnectorResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of the specified DICOM Service.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static Output getDicomService(GetDicomServiceArgs args) {
return getDicomService(args, InvokeOptions.Empty);
}
/**
* Gets the properties of the specified DICOM Service.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static CompletableFuture getDicomServicePlain(GetDicomServicePlainArgs args) {
return getDicomServicePlain(args, InvokeOptions.Empty);
}
/**
* Gets the properties of the specified DICOM Service.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static Output getDicomService(GetDicomServiceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:healthcareapis:getDicomService", TypeShape.of(GetDicomServiceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of the specified DICOM Service.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static CompletableFuture getDicomServicePlain(GetDicomServicePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:healthcareapis:getDicomService", TypeShape.of(GetDicomServiceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of the specified FHIR Service.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static Output getFhirService(GetFhirServiceArgs args) {
return getFhirService(args, InvokeOptions.Empty);
}
/**
* Gets the properties of the specified FHIR Service.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static CompletableFuture getFhirServicePlain(GetFhirServicePlainArgs args) {
return getFhirServicePlain(args, InvokeOptions.Empty);
}
/**
* Gets the properties of the specified FHIR Service.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static Output getFhirService(GetFhirServiceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:healthcareapis:getFhirService", TypeShape.of(GetFhirServiceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of the specified FHIR Service.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static CompletableFuture getFhirServicePlain(GetFhirServicePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:healthcareapis:getFhirService", TypeShape.of(GetFhirServiceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of the specified IoT Connector.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static Output getIotConnector(GetIotConnectorArgs args) {
return getIotConnector(args, InvokeOptions.Empty);
}
/**
* Gets the properties of the specified IoT Connector.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static CompletableFuture getIotConnectorPlain(GetIotConnectorPlainArgs args) {
return getIotConnectorPlain(args, InvokeOptions.Empty);
}
/**
* Gets the properties of the specified IoT Connector.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static Output getIotConnector(GetIotConnectorArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:healthcareapis:getIotConnector", TypeShape.of(GetIotConnectorResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of the specified IoT Connector.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static CompletableFuture getIotConnectorPlain(GetIotConnectorPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:healthcareapis:getIotConnector", TypeShape.of(GetIotConnectorResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of the specified Iot Connector FHIR destination.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static Output getIotConnectorFhirDestination(GetIotConnectorFhirDestinationArgs args) {
return getIotConnectorFhirDestination(args, InvokeOptions.Empty);
}
/**
* Gets the properties of the specified Iot Connector FHIR destination.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static CompletableFuture getIotConnectorFhirDestinationPlain(GetIotConnectorFhirDestinationPlainArgs args) {
return getIotConnectorFhirDestinationPlain(args, InvokeOptions.Empty);
}
/**
* Gets the properties of the specified Iot Connector FHIR destination.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static Output getIotConnectorFhirDestination(GetIotConnectorFhirDestinationArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:healthcareapis:getIotConnectorFhirDestination", TypeShape.of(GetIotConnectorFhirDestinationResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of the specified Iot Connector FHIR destination.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static CompletableFuture getIotConnectorFhirDestinationPlain(GetIotConnectorFhirDestinationPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:healthcareapis:getIotConnectorFhirDestination", TypeShape.of(GetIotConnectorFhirDestinationResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified private endpoint connection associated with the service.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static Output getPrivateEndpointConnection(GetPrivateEndpointConnectionArgs args) {
return getPrivateEndpointConnection(args, InvokeOptions.Empty);
}
/**
* Gets the specified private endpoint connection associated with the service.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static CompletableFuture getPrivateEndpointConnectionPlain(GetPrivateEndpointConnectionPlainArgs args) {
return getPrivateEndpointConnectionPlain(args, InvokeOptions.Empty);
}
/**
* Gets the specified private endpoint connection associated with the service.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static Output getPrivateEndpointConnection(GetPrivateEndpointConnectionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:healthcareapis:getPrivateEndpointConnection", TypeShape.of(GetPrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified private endpoint connection associated with the service.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static CompletableFuture getPrivateEndpointConnectionPlain(GetPrivateEndpointConnectionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:healthcareapis:getPrivateEndpointConnection", TypeShape.of(GetPrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Get the metadata of a service instance.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2020-03-15, 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static Output getService(GetServiceArgs args) {
return getService(args, InvokeOptions.Empty);
}
/**
* Get the metadata of a service instance.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2020-03-15, 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static CompletableFuture getServicePlain(GetServicePlainArgs args) {
return getServicePlain(args, InvokeOptions.Empty);
}
/**
* Get the metadata of a service instance.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2020-03-15, 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static Output getService(GetServiceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:healthcareapis:getService", TypeShape.of(GetServiceResult.class), args, Utilities.withVersion(options));
}
/**
* Get the metadata of a service instance.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2020-03-15, 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static CompletableFuture getServicePlain(GetServicePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:healthcareapis:getService", TypeShape.of(GetServiceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of the specified workspace.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static Output getWorkspace(GetWorkspaceArgs args) {
return getWorkspace(args, InvokeOptions.Empty);
}
/**
* Gets the properties of the specified workspace.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static CompletableFuture getWorkspacePlain(GetWorkspacePlainArgs args) {
return getWorkspacePlain(args, InvokeOptions.Empty);
}
/**
* Gets the properties of the specified workspace.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static Output getWorkspace(GetWorkspaceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:healthcareapis:getWorkspace", TypeShape.of(GetWorkspaceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the properties of the specified workspace.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static CompletableFuture getWorkspacePlain(GetWorkspacePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:healthcareapis:getWorkspace", TypeShape.of(GetWorkspaceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified private endpoint connection associated with the workspace.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static Output getWorkspacePrivateEndpointConnection(GetWorkspacePrivateEndpointConnectionArgs args) {
return getWorkspacePrivateEndpointConnection(args, InvokeOptions.Empty);
}
/**
* Gets the specified private endpoint connection associated with the workspace.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static CompletableFuture getWorkspacePrivateEndpointConnectionPlain(GetWorkspacePrivateEndpointConnectionPlainArgs args) {
return getWorkspacePrivateEndpointConnectionPlain(args, InvokeOptions.Empty);
}
/**
* Gets the specified private endpoint connection associated with the workspace.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static Output getWorkspacePrivateEndpointConnection(GetWorkspacePrivateEndpointConnectionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:healthcareapis:getWorkspacePrivateEndpointConnection", TypeShape.of(GetWorkspacePrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified private endpoint connection associated with the workspace.
* Azure REST API version: 2023-02-28.
*
* Other available API versions: 2023-09-06, 2023-11-01, 2023-12-01, 2024-03-01, 2024-03-31.
*
*/
public static CompletableFuture getWorkspacePrivateEndpointConnectionPlain(GetWorkspacePrivateEndpointConnectionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:healthcareapis:getWorkspacePrivateEndpointConnection", TypeShape.of(GetWorkspacePrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy