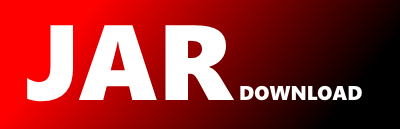
com.pulumi.azurenative.hybriddata.JobDefinitionArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.hybriddata;
import com.pulumi.azurenative.hybriddata.enums.RunLocation;
import com.pulumi.azurenative.hybriddata.enums.State;
import com.pulumi.azurenative.hybriddata.enums.UserConfirmation;
import com.pulumi.azurenative.hybriddata.inputs.CustomerSecretArgs;
import com.pulumi.azurenative.hybriddata.inputs.ScheduleArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class JobDefinitionArgs extends com.pulumi.resources.ResourceArgs {
public static final JobDefinitionArgs Empty = new JobDefinitionArgs();
/**
* List of customer secrets containing a key identifier and key value. The key identifier is a way for the specific data source to understand the key. Value contains customer secret encrypted by the encryptionKeys.
*
*/
@Import(name="customerSecrets")
private @Nullable Output> customerSecrets;
/**
* @return List of customer secrets containing a key identifier and key value. The key identifier is a way for the specific data source to understand the key. Value contains customer secret encrypted by the encryptionKeys.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy