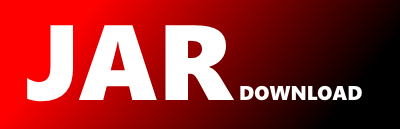
com.pulumi.azurenative.impact.outputs.InsightPropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.impact.outputs;
import com.pulumi.azurenative.impact.outputs.ContentResponse;
import com.pulumi.azurenative.impact.outputs.ImpactDetailsResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Object;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class InsightPropertiesResponse {
/**
* @return additional details of the insight.
*
*/
private @Nullable Object additionalDetails;
/**
* @return category of the insight.
*
*/
private String category;
/**
* @return Contains title & description for the insight
*
*/
private ContentResponse content;
/**
* @return Identifier of the event that has been correlated with this insight. This can be used to aggregate insights for the same event.
*
*/
private @Nullable String eventId;
/**
* @return Time of the event, which has been correlated the impact.
*
*/
private @Nullable String eventTime;
/**
* @return Identifier that can be used to group similar insights.
*
*/
private @Nullable String groupId;
/**
* @return details of of the impact for which insight has been generated.
*
*/
private ImpactDetailsResponse impact;
/**
* @return unique id of the insight.
*
*/
private String insightUniqueId;
/**
* @return Resource provisioning state.
*
*/
private String provisioningState;
/**
* @return status of the insight. example resolved, repaired, other.
*
*/
private @Nullable String status;
private InsightPropertiesResponse() {}
/**
* @return additional details of the insight.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy