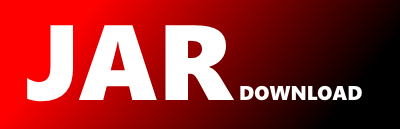
com.pulumi.azurenative.importexport.inputs.ShippingInformationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.importexport.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Contains information about the Microsoft datacenter to which the drives should be shipped.
*
*/
public final class ShippingInformationArgs extends com.pulumi.resources.ResourceArgs {
public static final ShippingInformationArgs Empty = new ShippingInformationArgs();
/**
* The city name to use when returning the drives.
*
*/
@Import(name="city")
private @Nullable Output city;
/**
* @return The city name to use when returning the drives.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy