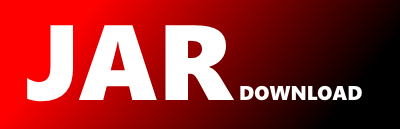
com.pulumi.azurenative.insights.ActivityLogAlertArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.insights;
import com.pulumi.azurenative.insights.inputs.ActionListArgs;
import com.pulumi.azurenative.insights.inputs.AlertRuleAllOfConditionArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ActivityLogAlertArgs extends com.pulumi.resources.ResourceArgs {
public static final ActivityLogAlertArgs Empty = new ActivityLogAlertArgs();
/**
* The actions that will activate when the condition is met.
*
*/
@Import(name="actions", required=true)
private Output actions;
/**
* @return The actions that will activate when the condition is met.
*
*/
public Output actions() {
return this.actions;
}
/**
* The name of the Activity Log Alert rule.
*
*/
@Import(name="activityLogAlertName")
private @Nullable Output activityLogAlertName;
/**
* @return The name of the Activity Log Alert rule.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy