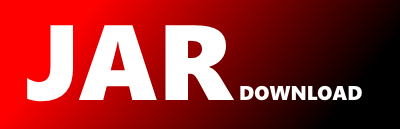
com.pulumi.azurenative.integrationspaces.InfrastructureResource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.integrationspaces;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.integrationspaces.InfrastructureResourceArgs;
import com.pulumi.azurenative.integrationspaces.outputs.SystemDataResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import javax.annotation.Nullable;
/**
* An infrastructure resource under Space.
* Azure REST API version: 2023-11-14-preview.
*
* ## Example Usage
* ### CreateOrUpdateInfrastructureResource
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.integrationspaces.InfrastructureResource;
* import com.pulumi.azurenative.integrationspaces.InfrastructureResourceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var infrastructureResource = new InfrastructureResource("infrastructureResource", InfrastructureResourceArgs.builder()
* .infrastructureResourceName("InfrastructureResource1")
* .resourceGroupName("testrg")
* .resourceId("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/testrg/providers/Microsoft.ApiManagement/service/APIM1")
* .resourceType("Microsoft.ApiManagement/service")
* .spaceName("Space1")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:integrationspaces:InfrastructureResource InfrastructureResource1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.IntegrationSpaces/spaces/{spaceName}/infrastructureResources/{infrastructureResourceName}
* ```
*
*/
@ResourceType(type="azure-native:integrationspaces:InfrastructureResource")
public class InfrastructureResource extends com.pulumi.resources.CustomResource {
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* The status of the last operation.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return The status of the last operation.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* The id of the infrastructure resource.
*
*/
@Export(name="resourceId", refs={String.class}, tree="[0]")
private Output resourceId;
/**
* @return The id of the infrastructure resource.
*
*/
public Output resourceId() {
return this.resourceId;
}
/**
* The type of the infrastructure resource.
*
*/
@Export(name="resourceType", refs={String.class}, tree="[0]")
private Output resourceType;
/**
* @return The type of the infrastructure resource.
*
*/
public Output resourceType() {
return this.resourceType;
}
/**
* Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public InfrastructureResource(java.lang.String name) {
this(name, InfrastructureResourceArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public InfrastructureResource(java.lang.String name, InfrastructureResourceArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public InfrastructureResource(java.lang.String name, InfrastructureResourceArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:integrationspaces:InfrastructureResource", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private InfrastructureResource(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:integrationspaces:InfrastructureResource", name, null, makeResourceOptions(options, id), false);
}
private static InfrastructureResourceArgs makeArgs(InfrastructureResourceArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? InfrastructureResourceArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:integrationspaces/v20231114preview:InfrastructureResource").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static InfrastructureResource get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new InfrastructureResource(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy