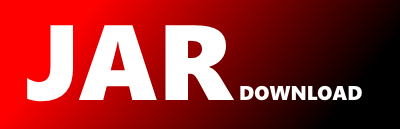
com.pulumi.azurenative.iotoperations.IotoperationsFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.iotoperations;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.iotoperations.inputs.GetBrokerArgs;
import com.pulumi.azurenative.iotoperations.inputs.GetBrokerAuthenticationArgs;
import com.pulumi.azurenative.iotoperations.inputs.GetBrokerAuthenticationPlainArgs;
import com.pulumi.azurenative.iotoperations.inputs.GetBrokerAuthorizationArgs;
import com.pulumi.azurenative.iotoperations.inputs.GetBrokerAuthorizationPlainArgs;
import com.pulumi.azurenative.iotoperations.inputs.GetBrokerListenerArgs;
import com.pulumi.azurenative.iotoperations.inputs.GetBrokerListenerPlainArgs;
import com.pulumi.azurenative.iotoperations.inputs.GetBrokerPlainArgs;
import com.pulumi.azurenative.iotoperations.inputs.GetDataFlowArgs;
import com.pulumi.azurenative.iotoperations.inputs.GetDataFlowEndpointArgs;
import com.pulumi.azurenative.iotoperations.inputs.GetDataFlowEndpointPlainArgs;
import com.pulumi.azurenative.iotoperations.inputs.GetDataFlowPlainArgs;
import com.pulumi.azurenative.iotoperations.inputs.GetDataFlowProfileArgs;
import com.pulumi.azurenative.iotoperations.inputs.GetDataFlowProfilePlainArgs;
import com.pulumi.azurenative.iotoperations.inputs.GetInstanceArgs;
import com.pulumi.azurenative.iotoperations.inputs.GetInstancePlainArgs;
import com.pulumi.azurenative.iotoperations.outputs.GetBrokerAuthenticationResult;
import com.pulumi.azurenative.iotoperations.outputs.GetBrokerAuthorizationResult;
import com.pulumi.azurenative.iotoperations.outputs.GetBrokerListenerResult;
import com.pulumi.azurenative.iotoperations.outputs.GetBrokerResult;
import com.pulumi.azurenative.iotoperations.outputs.GetDataFlowEndpointResult;
import com.pulumi.azurenative.iotoperations.outputs.GetDataFlowProfileResult;
import com.pulumi.azurenative.iotoperations.outputs.GetDataFlowResult;
import com.pulumi.azurenative.iotoperations.outputs.GetInstanceResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class IotoperationsFunctions {
/**
* Get a BrokerResource
* Azure REST API version: 2024-07-01-preview.
*
* Other available API versions: 2024-08-15-preview, 2024-09-15-preview, 2024-11-01.
*
*/
public static Output getBroker(GetBrokerArgs args) {
return getBroker(args, InvokeOptions.Empty);
}
/**
* Get a BrokerResource
* Azure REST API version: 2024-07-01-preview.
*
* Other available API versions: 2024-08-15-preview, 2024-09-15-preview, 2024-11-01.
*
*/
public static CompletableFuture getBrokerPlain(GetBrokerPlainArgs args) {
return getBrokerPlain(args, InvokeOptions.Empty);
}
/**
* Get a BrokerResource
* Azure REST API version: 2024-07-01-preview.
*
* Other available API versions: 2024-08-15-preview, 2024-09-15-preview, 2024-11-01.
*
*/
public static Output getBroker(GetBrokerArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:iotoperations:getBroker", TypeShape.of(GetBrokerResult.class), args, Utilities.withVersion(options));
}
/**
* Get a BrokerResource
* Azure REST API version: 2024-07-01-preview.
*
* Other available API versions: 2024-08-15-preview, 2024-09-15-preview, 2024-11-01.
*
*/
public static CompletableFuture getBrokerPlain(GetBrokerPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:iotoperations:getBroker", TypeShape.of(GetBrokerResult.class), args, Utilities.withVersion(options));
}
/**
* Get a BrokerAuthenticationResource
* Azure REST API version: 2024-07-01-preview.
*
* Other available API versions: 2024-08-15-preview, 2024-09-15-preview, 2024-11-01.
*
*/
public static Output getBrokerAuthentication(GetBrokerAuthenticationArgs args) {
return getBrokerAuthentication(args, InvokeOptions.Empty);
}
/**
* Get a BrokerAuthenticationResource
* Azure REST API version: 2024-07-01-preview.
*
* Other available API versions: 2024-08-15-preview, 2024-09-15-preview, 2024-11-01.
*
*/
public static CompletableFuture getBrokerAuthenticationPlain(GetBrokerAuthenticationPlainArgs args) {
return getBrokerAuthenticationPlain(args, InvokeOptions.Empty);
}
/**
* Get a BrokerAuthenticationResource
* Azure REST API version: 2024-07-01-preview.
*
* Other available API versions: 2024-08-15-preview, 2024-09-15-preview, 2024-11-01.
*
*/
public static Output getBrokerAuthentication(GetBrokerAuthenticationArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:iotoperations:getBrokerAuthentication", TypeShape.of(GetBrokerAuthenticationResult.class), args, Utilities.withVersion(options));
}
/**
* Get a BrokerAuthenticationResource
* Azure REST API version: 2024-07-01-preview.
*
* Other available API versions: 2024-08-15-preview, 2024-09-15-preview, 2024-11-01.
*
*/
public static CompletableFuture getBrokerAuthenticationPlain(GetBrokerAuthenticationPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:iotoperations:getBrokerAuthentication", TypeShape.of(GetBrokerAuthenticationResult.class), args, Utilities.withVersion(options));
}
/**
* Get a BrokerAuthorizationResource
* Azure REST API version: 2024-07-01-preview.
*
* Other available API versions: 2024-08-15-preview, 2024-09-15-preview, 2024-11-01.
*
*/
public static Output getBrokerAuthorization(GetBrokerAuthorizationArgs args) {
return getBrokerAuthorization(args, InvokeOptions.Empty);
}
/**
* Get a BrokerAuthorizationResource
* Azure REST API version: 2024-07-01-preview.
*
* Other available API versions: 2024-08-15-preview, 2024-09-15-preview, 2024-11-01.
*
*/
public static CompletableFuture getBrokerAuthorizationPlain(GetBrokerAuthorizationPlainArgs args) {
return getBrokerAuthorizationPlain(args, InvokeOptions.Empty);
}
/**
* Get a BrokerAuthorizationResource
* Azure REST API version: 2024-07-01-preview.
*
* Other available API versions: 2024-08-15-preview, 2024-09-15-preview, 2024-11-01.
*
*/
public static Output getBrokerAuthorization(GetBrokerAuthorizationArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:iotoperations:getBrokerAuthorization", TypeShape.of(GetBrokerAuthorizationResult.class), args, Utilities.withVersion(options));
}
/**
* Get a BrokerAuthorizationResource
* Azure REST API version: 2024-07-01-preview.
*
* Other available API versions: 2024-08-15-preview, 2024-09-15-preview, 2024-11-01.
*
*/
public static CompletableFuture getBrokerAuthorizationPlain(GetBrokerAuthorizationPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:iotoperations:getBrokerAuthorization", TypeShape.of(GetBrokerAuthorizationResult.class), args, Utilities.withVersion(options));
}
/**
* Get a BrokerListenerResource
* Azure REST API version: 2024-07-01-preview.
*
* Other available API versions: 2024-08-15-preview, 2024-09-15-preview, 2024-11-01.
*
*/
public static Output getBrokerListener(GetBrokerListenerArgs args) {
return getBrokerListener(args, InvokeOptions.Empty);
}
/**
* Get a BrokerListenerResource
* Azure REST API version: 2024-07-01-preview.
*
* Other available API versions: 2024-08-15-preview, 2024-09-15-preview, 2024-11-01.
*
*/
public static CompletableFuture getBrokerListenerPlain(GetBrokerListenerPlainArgs args) {
return getBrokerListenerPlain(args, InvokeOptions.Empty);
}
/**
* Get a BrokerListenerResource
* Azure REST API version: 2024-07-01-preview.
*
* Other available API versions: 2024-08-15-preview, 2024-09-15-preview, 2024-11-01.
*
*/
public static Output getBrokerListener(GetBrokerListenerArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:iotoperations:getBrokerListener", TypeShape.of(GetBrokerListenerResult.class), args, Utilities.withVersion(options));
}
/**
* Get a BrokerListenerResource
* Azure REST API version: 2024-07-01-preview.
*
* Other available API versions: 2024-08-15-preview, 2024-09-15-preview, 2024-11-01.
*
*/
public static CompletableFuture getBrokerListenerPlain(GetBrokerListenerPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:iotoperations:getBrokerListener", TypeShape.of(GetBrokerListenerResult.class), args, Utilities.withVersion(options));
}
/**
* Get a DataFlowResource
* Azure REST API version: 2024-07-01-preview.
*
*/
public static Output getDataFlow(GetDataFlowArgs args) {
return getDataFlow(args, InvokeOptions.Empty);
}
/**
* Get a DataFlowResource
* Azure REST API version: 2024-07-01-preview.
*
*/
public static CompletableFuture getDataFlowPlain(GetDataFlowPlainArgs args) {
return getDataFlowPlain(args, InvokeOptions.Empty);
}
/**
* Get a DataFlowResource
* Azure REST API version: 2024-07-01-preview.
*
*/
public static Output getDataFlow(GetDataFlowArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:iotoperations:getDataFlow", TypeShape.of(GetDataFlowResult.class), args, Utilities.withVersion(options));
}
/**
* Get a DataFlowResource
* Azure REST API version: 2024-07-01-preview.
*
*/
public static CompletableFuture getDataFlowPlain(GetDataFlowPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:iotoperations:getDataFlow", TypeShape.of(GetDataFlowResult.class), args, Utilities.withVersion(options));
}
/**
* Get a DataFlowEndpointResource
* Azure REST API version: 2024-07-01-preview.
*
*/
public static Output getDataFlowEndpoint(GetDataFlowEndpointArgs args) {
return getDataFlowEndpoint(args, InvokeOptions.Empty);
}
/**
* Get a DataFlowEndpointResource
* Azure REST API version: 2024-07-01-preview.
*
*/
public static CompletableFuture getDataFlowEndpointPlain(GetDataFlowEndpointPlainArgs args) {
return getDataFlowEndpointPlain(args, InvokeOptions.Empty);
}
/**
* Get a DataFlowEndpointResource
* Azure REST API version: 2024-07-01-preview.
*
*/
public static Output getDataFlowEndpoint(GetDataFlowEndpointArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:iotoperations:getDataFlowEndpoint", TypeShape.of(GetDataFlowEndpointResult.class), args, Utilities.withVersion(options));
}
/**
* Get a DataFlowEndpointResource
* Azure REST API version: 2024-07-01-preview.
*
*/
public static CompletableFuture getDataFlowEndpointPlain(GetDataFlowEndpointPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:iotoperations:getDataFlowEndpoint", TypeShape.of(GetDataFlowEndpointResult.class), args, Utilities.withVersion(options));
}
/**
* Get a DataFlowProfileResource
* Azure REST API version: 2024-07-01-preview.
*
*/
public static Output getDataFlowProfile(GetDataFlowProfileArgs args) {
return getDataFlowProfile(args, InvokeOptions.Empty);
}
/**
* Get a DataFlowProfileResource
* Azure REST API version: 2024-07-01-preview.
*
*/
public static CompletableFuture getDataFlowProfilePlain(GetDataFlowProfilePlainArgs args) {
return getDataFlowProfilePlain(args, InvokeOptions.Empty);
}
/**
* Get a DataFlowProfileResource
* Azure REST API version: 2024-07-01-preview.
*
*/
public static Output getDataFlowProfile(GetDataFlowProfileArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:iotoperations:getDataFlowProfile", TypeShape.of(GetDataFlowProfileResult.class), args, Utilities.withVersion(options));
}
/**
* Get a DataFlowProfileResource
* Azure REST API version: 2024-07-01-preview.
*
*/
public static CompletableFuture getDataFlowProfilePlain(GetDataFlowProfilePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:iotoperations:getDataFlowProfile", TypeShape.of(GetDataFlowProfileResult.class), args, Utilities.withVersion(options));
}
/**
* Get a InstanceResource
* Azure REST API version: 2024-07-01-preview.
*
* Other available API versions: 2024-08-15-preview, 2024-09-15-preview, 2024-11-01.
*
*/
public static Output getInstance(GetInstanceArgs args) {
return getInstance(args, InvokeOptions.Empty);
}
/**
* Get a InstanceResource
* Azure REST API version: 2024-07-01-preview.
*
* Other available API versions: 2024-08-15-preview, 2024-09-15-preview, 2024-11-01.
*
*/
public static CompletableFuture getInstancePlain(GetInstancePlainArgs args) {
return getInstancePlain(args, InvokeOptions.Empty);
}
/**
* Get a InstanceResource
* Azure REST API version: 2024-07-01-preview.
*
* Other available API versions: 2024-08-15-preview, 2024-09-15-preview, 2024-11-01.
*
*/
public static Output getInstance(GetInstanceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:iotoperations:getInstance", TypeShape.of(GetInstanceResult.class), args, Utilities.withVersion(options));
}
/**
* Get a InstanceResource
* Azure REST API version: 2024-07-01-preview.
*
* Other available API versions: 2024-08-15-preview, 2024-09-15-preview, 2024-11-01.
*
*/
public static CompletableFuture getInstancePlain(GetInstancePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:iotoperations:getInstance", TypeShape.of(GetInstanceResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy