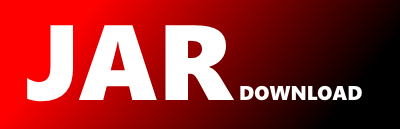
com.pulumi.azurenative.iotoperations.inputs.DataFlowEndpointMqttArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.iotoperations.inputs;
import com.pulumi.azurenative.iotoperations.enums.BrokerProtocolType;
import com.pulumi.azurenative.iotoperations.enums.MqttRetainType;
import com.pulumi.azurenative.iotoperations.inputs.TlsPropertiesArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Broker endpoint properties
*
*/
public final class DataFlowEndpointMqttArgs extends com.pulumi.resources.ResourceArgs {
public static final DataFlowEndpointMqttArgs Empty = new DataFlowEndpointMqttArgs();
/**
* Client ID prefix. Client ID generated by the dataflow is <prefix>-TBD. Optional; no prefix if omitted.
*
*/
@Import(name="clientIdPrefix")
private @Nullable Output clientIdPrefix;
/**
* @return Client ID prefix. Client ID generated by the dataflow is <prefix>-TBD. Optional; no prefix if omitted.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy