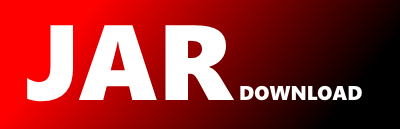
com.pulumi.azurenative.kubernetesruntime.KubernetesruntimeFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.kubernetesruntime;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.kubernetesruntime.inputs.GetBgpPeerArgs;
import com.pulumi.azurenative.kubernetesruntime.inputs.GetBgpPeerPlainArgs;
import com.pulumi.azurenative.kubernetesruntime.inputs.GetLoadBalancerArgs;
import com.pulumi.azurenative.kubernetesruntime.inputs.GetLoadBalancerPlainArgs;
import com.pulumi.azurenative.kubernetesruntime.inputs.GetServiceArgs;
import com.pulumi.azurenative.kubernetesruntime.inputs.GetServicePlainArgs;
import com.pulumi.azurenative.kubernetesruntime.inputs.GetStorageClassArgs;
import com.pulumi.azurenative.kubernetesruntime.inputs.GetStorageClassPlainArgs;
import com.pulumi.azurenative.kubernetesruntime.outputs.GetBgpPeerResult;
import com.pulumi.azurenative.kubernetesruntime.outputs.GetLoadBalancerResult;
import com.pulumi.azurenative.kubernetesruntime.outputs.GetServiceResult;
import com.pulumi.azurenative.kubernetesruntime.outputs.GetStorageClassResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class KubernetesruntimeFunctions {
/**
* Get a BgpPeer
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2023-10-01-preview.
*
*/
public static Output getBgpPeer(GetBgpPeerArgs args) {
return getBgpPeer(args, InvokeOptions.Empty);
}
/**
* Get a BgpPeer
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2023-10-01-preview.
*
*/
public static CompletableFuture getBgpPeerPlain(GetBgpPeerPlainArgs args) {
return getBgpPeerPlain(args, InvokeOptions.Empty);
}
/**
* Get a BgpPeer
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2023-10-01-preview.
*
*/
public static Output getBgpPeer(GetBgpPeerArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:kubernetesruntime:getBgpPeer", TypeShape.of(GetBgpPeerResult.class), args, Utilities.withVersion(options));
}
/**
* Get a BgpPeer
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2023-10-01-preview.
*
*/
public static CompletableFuture getBgpPeerPlain(GetBgpPeerPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:kubernetesruntime:getBgpPeer", TypeShape.of(GetBgpPeerResult.class), args, Utilities.withVersion(options));
}
/**
* Get a LoadBalancer
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2023-10-01-preview.
*
*/
public static Output getLoadBalancer(GetLoadBalancerArgs args) {
return getLoadBalancer(args, InvokeOptions.Empty);
}
/**
* Get a LoadBalancer
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2023-10-01-preview.
*
*/
public static CompletableFuture getLoadBalancerPlain(GetLoadBalancerPlainArgs args) {
return getLoadBalancerPlain(args, InvokeOptions.Empty);
}
/**
* Get a LoadBalancer
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2023-10-01-preview.
*
*/
public static Output getLoadBalancer(GetLoadBalancerArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:kubernetesruntime:getLoadBalancer", TypeShape.of(GetLoadBalancerResult.class), args, Utilities.withVersion(options));
}
/**
* Get a LoadBalancer
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2023-10-01-preview.
*
*/
public static CompletableFuture getLoadBalancerPlain(GetLoadBalancerPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:kubernetesruntime:getLoadBalancer", TypeShape.of(GetLoadBalancerResult.class), args, Utilities.withVersion(options));
}
/**
* Get a ServiceResource
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2023-10-01-preview.
*
*/
public static Output getService(GetServiceArgs args) {
return getService(args, InvokeOptions.Empty);
}
/**
* Get a ServiceResource
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2023-10-01-preview.
*
*/
public static CompletableFuture getServicePlain(GetServicePlainArgs args) {
return getServicePlain(args, InvokeOptions.Empty);
}
/**
* Get a ServiceResource
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2023-10-01-preview.
*
*/
public static Output getService(GetServiceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:kubernetesruntime:getService", TypeShape.of(GetServiceResult.class), args, Utilities.withVersion(options));
}
/**
* Get a ServiceResource
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2023-10-01-preview.
*
*/
public static CompletableFuture getServicePlain(GetServicePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:kubernetesruntime:getService", TypeShape.of(GetServiceResult.class), args, Utilities.withVersion(options));
}
/**
* Get a StorageClassResource
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2023-10-01-preview.
*
*/
public static Output getStorageClass(GetStorageClassArgs args) {
return getStorageClass(args, InvokeOptions.Empty);
}
/**
* Get a StorageClassResource
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2023-10-01-preview.
*
*/
public static CompletableFuture getStorageClassPlain(GetStorageClassPlainArgs args) {
return getStorageClassPlain(args, InvokeOptions.Empty);
}
/**
* Get a StorageClassResource
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2023-10-01-preview.
*
*/
public static Output getStorageClass(GetStorageClassArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:kubernetesruntime:getStorageClass", TypeShape.of(GetStorageClassResult.class), args, Utilities.withVersion(options));
}
/**
* Get a StorageClassResource
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2023-10-01-preview.
*
*/
public static CompletableFuture getStorageClassPlain(GetStorageClassPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:kubernetesruntime:getStorageClass", TypeShape.of(GetStorageClassResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy