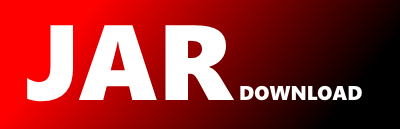
com.pulumi.azurenative.kusto.ClusterArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.kusto;
import com.pulumi.azurenative.kusto.enums.ClusterNetworkAccessFlag;
import com.pulumi.azurenative.kusto.enums.EngineType;
import com.pulumi.azurenative.kusto.enums.PublicIPType;
import com.pulumi.azurenative.kusto.enums.PublicNetworkAccess;
import com.pulumi.azurenative.kusto.inputs.AcceptedAudiencesArgs;
import com.pulumi.azurenative.kusto.inputs.AzureSkuArgs;
import com.pulumi.azurenative.kusto.inputs.IdentityArgs;
import com.pulumi.azurenative.kusto.inputs.KeyVaultPropertiesArgs;
import com.pulumi.azurenative.kusto.inputs.LanguageExtensionsListArgs;
import com.pulumi.azurenative.kusto.inputs.OptimizedAutoscaleArgs;
import com.pulumi.azurenative.kusto.inputs.TrustedExternalTenantArgs;
import com.pulumi.azurenative.kusto.inputs.VirtualNetworkConfigurationArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ClusterArgs extends com.pulumi.resources.ResourceArgs {
public static final ClusterArgs Empty = new ClusterArgs();
/**
* The cluster's accepted audiences.
*
*/
@Import(name="acceptedAudiences")
private @Nullable Output> acceptedAudiences;
/**
* @return The cluster's accepted audiences.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy