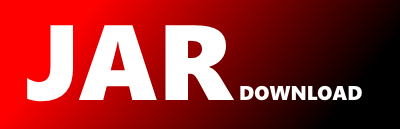
com.pulumi.azurenative.kusto.outputs.TableLevelSharingPropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.kusto.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import javax.annotation.Nullable;
@CustomType
public final class TableLevelSharingPropertiesResponse {
/**
* @return List of external tables to exclude from the follower database
*
*/
private @Nullable List externalTablesToExclude;
/**
* @return List of external tables to include in the follower database
*
*/
private @Nullable List externalTablesToInclude;
/**
* @return List of functions to exclude from the follower database
*
*/
private @Nullable List functionsToExclude;
/**
* @return List of functions to include in the follower database
*
*/
private @Nullable List functionsToInclude;
/**
* @return List of materialized views to exclude from the follower database
*
*/
private @Nullable List materializedViewsToExclude;
/**
* @return List of materialized views to include in the follower database
*
*/
private @Nullable List materializedViewsToInclude;
/**
* @return List of tables to exclude from the follower database
*
*/
private @Nullable List tablesToExclude;
/**
* @return List of tables to include in the follower database
*
*/
private @Nullable List tablesToInclude;
private TableLevelSharingPropertiesResponse() {}
/**
* @return List of external tables to exclude from the follower database
*
*/
public List externalTablesToExclude() {
return this.externalTablesToExclude == null ? List.of() : this.externalTablesToExclude;
}
/**
* @return List of external tables to include in the follower database
*
*/
public List externalTablesToInclude() {
return this.externalTablesToInclude == null ? List.of() : this.externalTablesToInclude;
}
/**
* @return List of functions to exclude from the follower database
*
*/
public List functionsToExclude() {
return this.functionsToExclude == null ? List.of() : this.functionsToExclude;
}
/**
* @return List of functions to include in the follower database
*
*/
public List functionsToInclude() {
return this.functionsToInclude == null ? List.of() : this.functionsToInclude;
}
/**
* @return List of materialized views to exclude from the follower database
*
*/
public List materializedViewsToExclude() {
return this.materializedViewsToExclude == null ? List.of() : this.materializedViewsToExclude;
}
/**
* @return List of materialized views to include in the follower database
*
*/
public List materializedViewsToInclude() {
return this.materializedViewsToInclude == null ? List.of() : this.materializedViewsToInclude;
}
/**
* @return List of tables to exclude from the follower database
*
*/
public List tablesToExclude() {
return this.tablesToExclude == null ? List.of() : this.tablesToExclude;
}
/**
* @return List of tables to include in the follower database
*
*/
public List tablesToInclude() {
return this.tablesToInclude == null ? List.of() : this.tablesToInclude;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(TableLevelSharingPropertiesResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List externalTablesToExclude;
private @Nullable List externalTablesToInclude;
private @Nullable List functionsToExclude;
private @Nullable List functionsToInclude;
private @Nullable List materializedViewsToExclude;
private @Nullable List materializedViewsToInclude;
private @Nullable List tablesToExclude;
private @Nullable List tablesToInclude;
public Builder() {}
public Builder(TableLevelSharingPropertiesResponse defaults) {
Objects.requireNonNull(defaults);
this.externalTablesToExclude = defaults.externalTablesToExclude;
this.externalTablesToInclude = defaults.externalTablesToInclude;
this.functionsToExclude = defaults.functionsToExclude;
this.functionsToInclude = defaults.functionsToInclude;
this.materializedViewsToExclude = defaults.materializedViewsToExclude;
this.materializedViewsToInclude = defaults.materializedViewsToInclude;
this.tablesToExclude = defaults.tablesToExclude;
this.tablesToInclude = defaults.tablesToInclude;
}
@CustomType.Setter
public Builder externalTablesToExclude(@Nullable List externalTablesToExclude) {
this.externalTablesToExclude = externalTablesToExclude;
return this;
}
public Builder externalTablesToExclude(String... externalTablesToExclude) {
return externalTablesToExclude(List.of(externalTablesToExclude));
}
@CustomType.Setter
public Builder externalTablesToInclude(@Nullable List externalTablesToInclude) {
this.externalTablesToInclude = externalTablesToInclude;
return this;
}
public Builder externalTablesToInclude(String... externalTablesToInclude) {
return externalTablesToInclude(List.of(externalTablesToInclude));
}
@CustomType.Setter
public Builder functionsToExclude(@Nullable List functionsToExclude) {
this.functionsToExclude = functionsToExclude;
return this;
}
public Builder functionsToExclude(String... functionsToExclude) {
return functionsToExclude(List.of(functionsToExclude));
}
@CustomType.Setter
public Builder functionsToInclude(@Nullable List functionsToInclude) {
this.functionsToInclude = functionsToInclude;
return this;
}
public Builder functionsToInclude(String... functionsToInclude) {
return functionsToInclude(List.of(functionsToInclude));
}
@CustomType.Setter
public Builder materializedViewsToExclude(@Nullable List materializedViewsToExclude) {
this.materializedViewsToExclude = materializedViewsToExclude;
return this;
}
public Builder materializedViewsToExclude(String... materializedViewsToExclude) {
return materializedViewsToExclude(List.of(materializedViewsToExclude));
}
@CustomType.Setter
public Builder materializedViewsToInclude(@Nullable List materializedViewsToInclude) {
this.materializedViewsToInclude = materializedViewsToInclude;
return this;
}
public Builder materializedViewsToInclude(String... materializedViewsToInclude) {
return materializedViewsToInclude(List.of(materializedViewsToInclude));
}
@CustomType.Setter
public Builder tablesToExclude(@Nullable List tablesToExclude) {
this.tablesToExclude = tablesToExclude;
return this;
}
public Builder tablesToExclude(String... tablesToExclude) {
return tablesToExclude(List.of(tablesToExclude));
}
@CustomType.Setter
public Builder tablesToInclude(@Nullable List tablesToInclude) {
this.tablesToInclude = tablesToInclude;
return this;
}
public Builder tablesToInclude(String... tablesToInclude) {
return tablesToInclude(List.of(tablesToInclude));
}
public TableLevelSharingPropertiesResponse build() {
final var _resultValue = new TableLevelSharingPropertiesResponse();
_resultValue.externalTablesToExclude = externalTablesToExclude;
_resultValue.externalTablesToInclude = externalTablesToInclude;
_resultValue.functionsToExclude = functionsToExclude;
_resultValue.functionsToInclude = functionsToInclude;
_resultValue.materializedViewsToExclude = materializedViewsToExclude;
_resultValue.materializedViewsToInclude = materializedViewsToInclude;
_resultValue.tablesToExclude = tablesToExclude;
_resultValue.tablesToInclude = tablesToInclude;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy