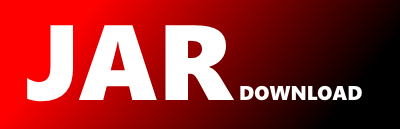
com.pulumi.azurenative.machinelearningservices.MachineLearningDatasetArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.machinelearningservices;
import com.pulumi.azurenative.machinelearningservices.enums.DatasetType;
import com.pulumi.azurenative.machinelearningservices.inputs.DatasetCreateRequestParametersArgs;
import com.pulumi.azurenative.machinelearningservices.inputs.DatasetCreateRequestRegistrationArgs;
import com.pulumi.azurenative.machinelearningservices.inputs.DatasetCreateRequestTimeSeriesArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class MachineLearningDatasetArgs extends com.pulumi.resources.ResourceArgs {
public static final MachineLearningDatasetArgs Empty = new MachineLearningDatasetArgs();
/**
* The Dataset name.
*
*/
@Import(name="datasetName")
private @Nullable Output datasetName;
/**
* @return The Dataset name.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy