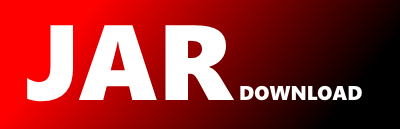
com.pulumi.azurenative.machinelearningservices.outputs.EnvironmentVersionResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.machinelearningservices.outputs;
import com.pulumi.azurenative.machinelearningservices.outputs.BuildContextResponse;
import com.pulumi.azurenative.machinelearningservices.outputs.InferenceContainerPropertiesResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class EnvironmentVersionResponse {
/**
* @return Defines if image needs to be rebuilt based on base image changes.
*
*/
private @Nullable String autoRebuild;
/**
* @return Configuration settings for Docker build context.
*
*/
private @Nullable BuildContextResponse build;
/**
* @return Standard configuration file used by Conda that lets you install any kind of package, including Python, R, and C/C++ packages.
* <see href="https://repo2docker.readthedocs.io/en/latest/config_files.html#environment-yml-install-a-conda-environment" />
*
*/
private @Nullable String condaFile;
/**
* @return The asset description text.
*
*/
private @Nullable String description;
/**
* @return Environment type is either user managed or curated by the Azure ML service
* <see href="https://docs.microsoft.com/en-us/azure/machine-learning/resource-curated-environments" />
*
*/
private String environmentType;
/**
* @return Name of the image that will be used for the environment.
* <seealso href="https://docs.microsoft.com/en-us/azure/machine-learning/how-to-deploy-custom-docker-image#use-a-custom-base-image" />
*
*/
private @Nullable String image;
/**
* @return Defines configuration specific to inference.
*
*/
private @Nullable InferenceContainerPropertiesResponse inferenceConfig;
/**
* @return If the name version are system generated (anonymous registration).
*
*/
private @Nullable Boolean isAnonymous;
/**
* @return Is the asset archived?
*
*/
private @Nullable Boolean isArchived;
/**
* @return The OS type of the environment.
*
*/
private @Nullable String osType;
/**
* @return The asset property dictionary.
*
*/
private @Nullable Map properties;
/**
* @return Provisioning state for the environment version.
*
*/
private String provisioningState;
/**
* @return Stage in the environment lifecycle assigned to this environment
*
*/
private @Nullable String stage;
/**
* @return Tag dictionary. Tags can be added, removed, and updated.
*
*/
private @Nullable Map tags;
private EnvironmentVersionResponse() {}
/**
* @return Defines if image needs to be rebuilt based on base image changes.
*
*/
public Optional autoRebuild() {
return Optional.ofNullable(this.autoRebuild);
}
/**
* @return Configuration settings for Docker build context.
*
*/
public Optional build() {
return Optional.ofNullable(this.build);
}
/**
* @return Standard configuration file used by Conda that lets you install any kind of package, including Python, R, and C/C++ packages.
* <see href="https://repo2docker.readthedocs.io/en/latest/config_files.html#environment-yml-install-a-conda-environment" />
*
*/
public Optional condaFile() {
return Optional.ofNullable(this.condaFile);
}
/**
* @return The asset description text.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return Environment type is either user managed or curated by the Azure ML service
* <see href="https://docs.microsoft.com/en-us/azure/machine-learning/resource-curated-environments" />
*
*/
public String environmentType() {
return this.environmentType;
}
/**
* @return Name of the image that will be used for the environment.
* <seealso href="https://docs.microsoft.com/en-us/azure/machine-learning/how-to-deploy-custom-docker-image#use-a-custom-base-image" />
*
*/
public Optional image() {
return Optional.ofNullable(this.image);
}
/**
* @return Defines configuration specific to inference.
*
*/
public Optional inferenceConfig() {
return Optional.ofNullable(this.inferenceConfig);
}
/**
* @return If the name version are system generated (anonymous registration).
*
*/
public Optional isAnonymous() {
return Optional.ofNullable(this.isAnonymous);
}
/**
* @return Is the asset archived?
*
*/
public Optional isArchived() {
return Optional.ofNullable(this.isArchived);
}
/**
* @return The OS type of the environment.
*
*/
public Optional osType() {
return Optional.ofNullable(this.osType);
}
/**
* @return The asset property dictionary.
*
*/
public Map properties() {
return this.properties == null ? Map.of() : this.properties;
}
/**
* @return Provisioning state for the environment version.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Stage in the environment lifecycle assigned to this environment
*
*/
public Optional stage() {
return Optional.ofNullable(this.stage);
}
/**
* @return Tag dictionary. Tags can be added, removed, and updated.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(EnvironmentVersionResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String autoRebuild;
private @Nullable BuildContextResponse build;
private @Nullable String condaFile;
private @Nullable String description;
private String environmentType;
private @Nullable String image;
private @Nullable InferenceContainerPropertiesResponse inferenceConfig;
private @Nullable Boolean isAnonymous;
private @Nullable Boolean isArchived;
private @Nullable String osType;
private @Nullable Map properties;
private String provisioningState;
private @Nullable String stage;
private @Nullable Map tags;
public Builder() {}
public Builder(EnvironmentVersionResponse defaults) {
Objects.requireNonNull(defaults);
this.autoRebuild = defaults.autoRebuild;
this.build = defaults.build;
this.condaFile = defaults.condaFile;
this.description = defaults.description;
this.environmentType = defaults.environmentType;
this.image = defaults.image;
this.inferenceConfig = defaults.inferenceConfig;
this.isAnonymous = defaults.isAnonymous;
this.isArchived = defaults.isArchived;
this.osType = defaults.osType;
this.properties = defaults.properties;
this.provisioningState = defaults.provisioningState;
this.stage = defaults.stage;
this.tags = defaults.tags;
}
@CustomType.Setter
public Builder autoRebuild(@Nullable String autoRebuild) {
this.autoRebuild = autoRebuild;
return this;
}
@CustomType.Setter
public Builder build(@Nullable BuildContextResponse build) {
this.build = build;
return this;
}
@CustomType.Setter
public Builder condaFile(@Nullable String condaFile) {
this.condaFile = condaFile;
return this;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder environmentType(String environmentType) {
if (environmentType == null) {
throw new MissingRequiredPropertyException("EnvironmentVersionResponse", "environmentType");
}
this.environmentType = environmentType;
return this;
}
@CustomType.Setter
public Builder image(@Nullable String image) {
this.image = image;
return this;
}
@CustomType.Setter
public Builder inferenceConfig(@Nullable InferenceContainerPropertiesResponse inferenceConfig) {
this.inferenceConfig = inferenceConfig;
return this;
}
@CustomType.Setter
public Builder isAnonymous(@Nullable Boolean isAnonymous) {
this.isAnonymous = isAnonymous;
return this;
}
@CustomType.Setter
public Builder isArchived(@Nullable Boolean isArchived) {
this.isArchived = isArchived;
return this;
}
@CustomType.Setter
public Builder osType(@Nullable String osType) {
this.osType = osType;
return this;
}
@CustomType.Setter
public Builder properties(@Nullable Map properties) {
this.properties = properties;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("EnvironmentVersionResponse", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder stage(@Nullable String stage) {
this.stage = stage;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
public EnvironmentVersionResponse build() {
final var _resultValue = new EnvironmentVersionResponse();
_resultValue.autoRebuild = autoRebuild;
_resultValue.build = build;
_resultValue.condaFile = condaFile;
_resultValue.description = description;
_resultValue.environmentType = environmentType;
_resultValue.image = image;
_resultValue.inferenceConfig = inferenceConfig;
_resultValue.isAnonymous = isAnonymous;
_resultValue.isArchived = isArchived;
_resultValue.osType = osType;
_resultValue.properties = properties;
_resultValue.provisioningState = provisioningState;
_resultValue.stage = stage;
_resultValue.tags = tags;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy