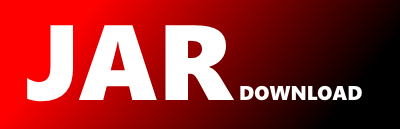
com.pulumi.azurenative.managednetworkfabric.inputs.IpPrefixPropertiesIpPrefixRulesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.managednetworkfabric.inputs;
import com.pulumi.azurenative.managednetworkfabric.enums.CommunityActionTypes;
import com.pulumi.azurenative.managednetworkfabric.enums.Condition;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class IpPrefixPropertiesIpPrefixRulesArgs extends com.pulumi.resources.ResourceArgs {
public static final IpPrefixPropertiesIpPrefixRulesArgs Empty = new IpPrefixPropertiesIpPrefixRulesArgs();
/**
* Action to be taken on the configuration. Example: Permit | Deny.
*
*/
@Import(name="action", required=true)
private Output> action;
/**
* @return Action to be taken on the configuration. Example: Permit | Deny.
*
*/
public Output> action() {
return this.action;
}
/**
* Specify prefix-list bounds.
*
*/
@Import(name="condition")
private @Nullable Output> condition;
/**
* @return Specify prefix-list bounds.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy