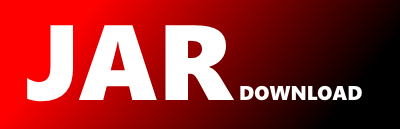
com.pulumi.azurenative.management.outputs.GetHierarchySettingResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.management.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetHierarchySettingResult {
/**
* @return Settings that sets the default Management Group under which new subscriptions get added in this tenant. For example, /providers/Microsoft.Management/managementGroups/defaultGroup
*
*/
private @Nullable String defaultManagementGroup;
/**
* @return The fully qualified ID for the settings object. For example, /providers/Microsoft.Management/managementGroups/0000000-0000-0000-0000-000000000000/settings/default.
*
*/
private String id;
/**
* @return The name of the object. In this case, default.
*
*/
private String name;
/**
* @return Indicates whether RBAC access is required upon group creation under the root Management Group. If set to true, user will require Microsoft.Management/managementGroups/write action on the root Management Group scope in order to create new Groups directly under the root. This will prevent new users from creating new Management Groups, unless they are given access.
*
*/
private @Nullable Boolean requireAuthorizationForGroupCreation;
/**
* @return The AAD Tenant ID associated with the hierarchy settings. For example, 00000000-0000-0000-0000-000000000000
*
*/
private @Nullable String tenantId;
/**
* @return The type of the resource. For example, Microsoft.Management/managementGroups/settings.
*
*/
private String type;
private GetHierarchySettingResult() {}
/**
* @return Settings that sets the default Management Group under which new subscriptions get added in this tenant. For example, /providers/Microsoft.Management/managementGroups/defaultGroup
*
*/
public Optional defaultManagementGroup() {
return Optional.ofNullable(this.defaultManagementGroup);
}
/**
* @return The fully qualified ID for the settings object. For example, /providers/Microsoft.Management/managementGroups/0000000-0000-0000-0000-000000000000/settings/default.
*
*/
public String id() {
return this.id;
}
/**
* @return The name of the object. In this case, default.
*
*/
public String name() {
return this.name;
}
/**
* @return Indicates whether RBAC access is required upon group creation under the root Management Group. If set to true, user will require Microsoft.Management/managementGroups/write action on the root Management Group scope in order to create new Groups directly under the root. This will prevent new users from creating new Management Groups, unless they are given access.
*
*/
public Optional requireAuthorizationForGroupCreation() {
return Optional.ofNullable(this.requireAuthorizationForGroupCreation);
}
/**
* @return The AAD Tenant ID associated with the hierarchy settings. For example, 00000000-0000-0000-0000-000000000000
*
*/
public Optional tenantId() {
return Optional.ofNullable(this.tenantId);
}
/**
* @return The type of the resource. For example, Microsoft.Management/managementGroups/settings.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetHierarchySettingResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String defaultManagementGroup;
private String id;
private String name;
private @Nullable Boolean requireAuthorizationForGroupCreation;
private @Nullable String tenantId;
private String type;
public Builder() {}
public Builder(GetHierarchySettingResult defaults) {
Objects.requireNonNull(defaults);
this.defaultManagementGroup = defaults.defaultManagementGroup;
this.id = defaults.id;
this.name = defaults.name;
this.requireAuthorizationForGroupCreation = defaults.requireAuthorizationForGroupCreation;
this.tenantId = defaults.tenantId;
this.type = defaults.type;
}
@CustomType.Setter
public Builder defaultManagementGroup(@Nullable String defaultManagementGroup) {
this.defaultManagementGroup = defaultManagementGroup;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetHierarchySettingResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetHierarchySettingResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder requireAuthorizationForGroupCreation(@Nullable Boolean requireAuthorizationForGroupCreation) {
this.requireAuthorizationForGroupCreation = requireAuthorizationForGroupCreation;
return this;
}
@CustomType.Setter
public Builder tenantId(@Nullable String tenantId) {
this.tenantId = tenantId;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetHierarchySettingResult", "type");
}
this.type = type;
return this;
}
public GetHierarchySettingResult build() {
final var _resultValue = new GetHierarchySettingResult();
_resultValue.defaultManagementGroup = defaultManagementGroup;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.requireAuthorizationForGroupCreation = requireAuthorizationForGroupCreation;
_resultValue.tenantId = tenantId;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy