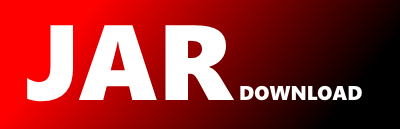
com.pulumi.azurenative.marketplace.outputs.GetPrivateStoreCollectionResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.marketplace.outputs;
import com.pulumi.azurenative.marketplace.outputs.RuleResponse;
import com.pulumi.azurenative.marketplace.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetPrivateStoreCollectionResult {
/**
* @return Indicating whether all subscriptions are selected (=true) or not (=false).
*
*/
private @Nullable Boolean allSubscriptions;
/**
* @return Gets list of collection rules
*
*/
private List appliedRules;
/**
* @return Indicating whether all items are approved for this collection (=true) or not (=false).
*
*/
private Boolean approveAllItems;
/**
* @return Gets the modified date of all items approved.
*
*/
private String approveAllItemsModifiedAt;
/**
* @return Gets or sets the association with Commercial's Billing Account.
*
*/
private @Nullable String claim;
/**
* @return Gets collection Id.
*
*/
private String collectionId;
/**
* @return Gets or sets collection name.
*
*/
private @Nullable String collectionName;
/**
* @return Indicating whether the collection is enabled or disabled.
*
*/
private @Nullable Boolean enabled;
/**
* @return The resource ID.
*
*/
private String id;
/**
* @return The name of the resource.
*
*/
private String name;
/**
* @return Gets the number of offers associated with the collection.
*
*/
private Double numberOfOffers;
/**
* @return Gets or sets subscription ids list. Empty list indicates all subscriptions are selected, null indicates no update is done, explicit list indicates the explicit selected subscriptions. On insert, null is considered as bad request
*
*/
private @Nullable List subscriptionsList;
/**
* @return Metadata pertaining to creation and last modification of the resource
*
*/
private SystemDataResponse systemData;
/**
* @return The type of the resource.
*
*/
private String type;
private GetPrivateStoreCollectionResult() {}
/**
* @return Indicating whether all subscriptions are selected (=true) or not (=false).
*
*/
public Optional allSubscriptions() {
return Optional.ofNullable(this.allSubscriptions);
}
/**
* @return Gets list of collection rules
*
*/
public List appliedRules() {
return this.appliedRules;
}
/**
* @return Indicating whether all items are approved for this collection (=true) or not (=false).
*
*/
public Boolean approveAllItems() {
return this.approveAllItems;
}
/**
* @return Gets the modified date of all items approved.
*
*/
public String approveAllItemsModifiedAt() {
return this.approveAllItemsModifiedAt;
}
/**
* @return Gets or sets the association with Commercial's Billing Account.
*
*/
public Optional claim() {
return Optional.ofNullable(this.claim);
}
/**
* @return Gets collection Id.
*
*/
public String collectionId() {
return this.collectionId;
}
/**
* @return Gets or sets collection name.
*
*/
public Optional collectionName() {
return Optional.ofNullable(this.collectionName);
}
/**
* @return Indicating whether the collection is enabled or disabled.
*
*/
public Optional enabled() {
return Optional.ofNullable(this.enabled);
}
/**
* @return The resource ID.
*
*/
public String id() {
return this.id;
}
/**
* @return The name of the resource.
*
*/
public String name() {
return this.name;
}
/**
* @return Gets the number of offers associated with the collection.
*
*/
public Double numberOfOffers() {
return this.numberOfOffers;
}
/**
* @return Gets or sets subscription ids list. Empty list indicates all subscriptions are selected, null indicates no update is done, explicit list indicates the explicit selected subscriptions. On insert, null is considered as bad request
*
*/
public List subscriptionsList() {
return this.subscriptionsList == null ? List.of() : this.subscriptionsList;
}
/**
* @return Metadata pertaining to creation and last modification of the resource
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return The type of the resource.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetPrivateStoreCollectionResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean allSubscriptions;
private List appliedRules;
private Boolean approveAllItems;
private String approveAllItemsModifiedAt;
private @Nullable String claim;
private String collectionId;
private @Nullable String collectionName;
private @Nullable Boolean enabled;
private String id;
private String name;
private Double numberOfOffers;
private @Nullable List subscriptionsList;
private SystemDataResponse systemData;
private String type;
public Builder() {}
public Builder(GetPrivateStoreCollectionResult defaults) {
Objects.requireNonNull(defaults);
this.allSubscriptions = defaults.allSubscriptions;
this.appliedRules = defaults.appliedRules;
this.approveAllItems = defaults.approveAllItems;
this.approveAllItemsModifiedAt = defaults.approveAllItemsModifiedAt;
this.claim = defaults.claim;
this.collectionId = defaults.collectionId;
this.collectionName = defaults.collectionName;
this.enabled = defaults.enabled;
this.id = defaults.id;
this.name = defaults.name;
this.numberOfOffers = defaults.numberOfOffers;
this.subscriptionsList = defaults.subscriptionsList;
this.systemData = defaults.systemData;
this.type = defaults.type;
}
@CustomType.Setter
public Builder allSubscriptions(@Nullable Boolean allSubscriptions) {
this.allSubscriptions = allSubscriptions;
return this;
}
@CustomType.Setter
public Builder appliedRules(List appliedRules) {
if (appliedRules == null) {
throw new MissingRequiredPropertyException("GetPrivateStoreCollectionResult", "appliedRules");
}
this.appliedRules = appliedRules;
return this;
}
public Builder appliedRules(RuleResponse... appliedRules) {
return appliedRules(List.of(appliedRules));
}
@CustomType.Setter
public Builder approveAllItems(Boolean approveAllItems) {
if (approveAllItems == null) {
throw new MissingRequiredPropertyException("GetPrivateStoreCollectionResult", "approveAllItems");
}
this.approveAllItems = approveAllItems;
return this;
}
@CustomType.Setter
public Builder approveAllItemsModifiedAt(String approveAllItemsModifiedAt) {
if (approveAllItemsModifiedAt == null) {
throw new MissingRequiredPropertyException("GetPrivateStoreCollectionResult", "approveAllItemsModifiedAt");
}
this.approveAllItemsModifiedAt = approveAllItemsModifiedAt;
return this;
}
@CustomType.Setter
public Builder claim(@Nullable String claim) {
this.claim = claim;
return this;
}
@CustomType.Setter
public Builder collectionId(String collectionId) {
if (collectionId == null) {
throw new MissingRequiredPropertyException("GetPrivateStoreCollectionResult", "collectionId");
}
this.collectionId = collectionId;
return this;
}
@CustomType.Setter
public Builder collectionName(@Nullable String collectionName) {
this.collectionName = collectionName;
return this;
}
@CustomType.Setter
public Builder enabled(@Nullable Boolean enabled) {
this.enabled = enabled;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetPrivateStoreCollectionResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetPrivateStoreCollectionResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder numberOfOffers(Double numberOfOffers) {
if (numberOfOffers == null) {
throw new MissingRequiredPropertyException("GetPrivateStoreCollectionResult", "numberOfOffers");
}
this.numberOfOffers = numberOfOffers;
return this;
}
@CustomType.Setter
public Builder subscriptionsList(@Nullable List subscriptionsList) {
this.subscriptionsList = subscriptionsList;
return this;
}
public Builder subscriptionsList(String... subscriptionsList) {
return subscriptionsList(List.of(subscriptionsList));
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetPrivateStoreCollectionResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetPrivateStoreCollectionResult", "type");
}
this.type = type;
return this;
}
public GetPrivateStoreCollectionResult build() {
final var _resultValue = new GetPrivateStoreCollectionResult();
_resultValue.allSubscriptions = allSubscriptions;
_resultValue.appliedRules = appliedRules;
_resultValue.approveAllItems = approveAllItems;
_resultValue.approveAllItemsModifiedAt = approveAllItemsModifiedAt;
_resultValue.claim = claim;
_resultValue.collectionId = collectionId;
_resultValue.collectionName = collectionName;
_resultValue.enabled = enabled;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.numberOfOffers = numberOfOffers;
_resultValue.subscriptionsList = subscriptionsList;
_resultValue.systemData = systemData;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy