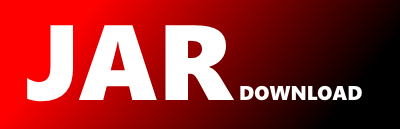
com.pulumi.azurenative.media.inputs.ContentKeyPolicyFairPlayConfigurationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.media.inputs;
import com.pulumi.azurenative.media.enums.ContentKeyPolicyFairPlayRentalAndLeaseKeyType;
import com.pulumi.azurenative.media.inputs.ContentKeyPolicyFairPlayOfflineRentalConfigurationArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Specifies a configuration for FairPlay licenses.
*
*/
public final class ContentKeyPolicyFairPlayConfigurationArgs extends com.pulumi.resources.ResourceArgs {
public static final ContentKeyPolicyFairPlayConfigurationArgs Empty = new ContentKeyPolicyFairPlayConfigurationArgs();
/**
* The key that must be used as FairPlay Application Secret key. This needs to be base64 encoded.
*
*/
@Import(name="ask", required=true)
private Output ask;
/**
* @return The key that must be used as FairPlay Application Secret key. This needs to be base64 encoded.
*
*/
public Output ask() {
return this.ask;
}
/**
* The Base64 representation of FairPlay certificate in PKCS 12 (pfx) format (including private key).
*
*/
@Import(name="fairPlayPfx", required=true)
private Output fairPlayPfx;
/**
* @return The Base64 representation of FairPlay certificate in PKCS 12 (pfx) format (including private key).
*
*/
public Output fairPlayPfx() {
return this.fairPlayPfx;
}
/**
* The password encrypting FairPlay certificate in PKCS 12 (pfx) format.
*
*/
@Import(name="fairPlayPfxPassword", required=true)
private Output fairPlayPfxPassword;
/**
* @return The password encrypting FairPlay certificate in PKCS 12 (pfx) format.
*
*/
public Output fairPlayPfxPassword() {
return this.fairPlayPfxPassword;
}
/**
* The discriminator for derived types.
* Expected value is '#Microsoft.Media.ContentKeyPolicyFairPlayConfiguration'.
*
*/
@Import(name="odataType", required=true)
private Output odataType;
/**
* @return The discriminator for derived types.
* Expected value is '#Microsoft.Media.ContentKeyPolicyFairPlayConfiguration'.
*
*/
public Output odataType() {
return this.odataType;
}
/**
* Offline rental policy
*
*/
@Import(name="offlineRentalConfiguration")
private @Nullable Output offlineRentalConfiguration;
/**
* @return Offline rental policy
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy