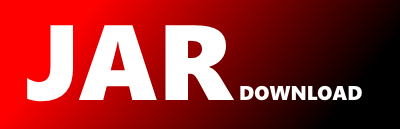
com.pulumi.azurenative.media.outputs.H264VideoResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.media.outputs;
import com.pulumi.azurenative.media.outputs.H264LayerResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class H264VideoResponse {
/**
* @return Tells the encoder how to choose its encoding settings. The default value is Balanced.
*
*/
private @Nullable String complexity;
/**
* @return The distance between two key frames. The value should be non-zero in the range [0.5, 20] seconds, specified in ISO 8601 format. The default is 2 seconds(PT2S). Note that this setting is ignored if VideoSyncMode.Passthrough is set, where the KeyFrameInterval value will follow the input source setting.
*
*/
private @Nullable String keyFrameInterval;
/**
* @return An optional label for the codec. The label can be used to control muxing behavior.
*
*/
private @Nullable String label;
/**
* @return The collection of output H.264 layers to be produced by the encoder.
*
*/
private @Nullable List layers;
/**
* @return The discriminator for derived types.
* Expected value is '#Microsoft.Media.H264Video'.
*
*/
private String odataType;
/**
* @return The video rate control mode
*
*/
private @Nullable String rateControlMode;
/**
* @return Whether or not the encoder should insert key frames at scene changes. If not specified, the default is false. This flag should be set to true only when the encoder is being configured to produce a single output video.
*
*/
private @Nullable Boolean sceneChangeDetection;
/**
* @return The resizing mode - how the input video will be resized to fit the desired output resolution(s). Default is AutoSize
*
*/
private @Nullable String stretchMode;
/**
* @return The Video Sync Mode
*
*/
private @Nullable String syncMode;
private H264VideoResponse() {}
/**
* @return Tells the encoder how to choose its encoding settings. The default value is Balanced.
*
*/
public Optional complexity() {
return Optional.ofNullable(this.complexity);
}
/**
* @return The distance between two key frames. The value should be non-zero in the range [0.5, 20] seconds, specified in ISO 8601 format. The default is 2 seconds(PT2S). Note that this setting is ignored if VideoSyncMode.Passthrough is set, where the KeyFrameInterval value will follow the input source setting.
*
*/
public Optional keyFrameInterval() {
return Optional.ofNullable(this.keyFrameInterval);
}
/**
* @return An optional label for the codec. The label can be used to control muxing behavior.
*
*/
public Optional label() {
return Optional.ofNullable(this.label);
}
/**
* @return The collection of output H.264 layers to be produced by the encoder.
*
*/
public List layers() {
return this.layers == null ? List.of() : this.layers;
}
/**
* @return The discriminator for derived types.
* Expected value is '#Microsoft.Media.H264Video'.
*
*/
public String odataType() {
return this.odataType;
}
/**
* @return The video rate control mode
*
*/
public Optional rateControlMode() {
return Optional.ofNullable(this.rateControlMode);
}
/**
* @return Whether or not the encoder should insert key frames at scene changes. If not specified, the default is false. This flag should be set to true only when the encoder is being configured to produce a single output video.
*
*/
public Optional sceneChangeDetection() {
return Optional.ofNullable(this.sceneChangeDetection);
}
/**
* @return The resizing mode - how the input video will be resized to fit the desired output resolution(s). Default is AutoSize
*
*/
public Optional stretchMode() {
return Optional.ofNullable(this.stretchMode);
}
/**
* @return The Video Sync Mode
*
*/
public Optional syncMode() {
return Optional.ofNullable(this.syncMode);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(H264VideoResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String complexity;
private @Nullable String keyFrameInterval;
private @Nullable String label;
private @Nullable List layers;
private String odataType;
private @Nullable String rateControlMode;
private @Nullable Boolean sceneChangeDetection;
private @Nullable String stretchMode;
private @Nullable String syncMode;
public Builder() {}
public Builder(H264VideoResponse defaults) {
Objects.requireNonNull(defaults);
this.complexity = defaults.complexity;
this.keyFrameInterval = defaults.keyFrameInterval;
this.label = defaults.label;
this.layers = defaults.layers;
this.odataType = defaults.odataType;
this.rateControlMode = defaults.rateControlMode;
this.sceneChangeDetection = defaults.sceneChangeDetection;
this.stretchMode = defaults.stretchMode;
this.syncMode = defaults.syncMode;
}
@CustomType.Setter
public Builder complexity(@Nullable String complexity) {
this.complexity = complexity;
return this;
}
@CustomType.Setter
public Builder keyFrameInterval(@Nullable String keyFrameInterval) {
this.keyFrameInterval = keyFrameInterval;
return this;
}
@CustomType.Setter
public Builder label(@Nullable String label) {
this.label = label;
return this;
}
@CustomType.Setter
public Builder layers(@Nullable List layers) {
this.layers = layers;
return this;
}
public Builder layers(H264LayerResponse... layers) {
return layers(List.of(layers));
}
@CustomType.Setter
public Builder odataType(String odataType) {
if (odataType == null) {
throw new MissingRequiredPropertyException("H264VideoResponse", "odataType");
}
this.odataType = odataType;
return this;
}
@CustomType.Setter
public Builder rateControlMode(@Nullable String rateControlMode) {
this.rateControlMode = rateControlMode;
return this;
}
@CustomType.Setter
public Builder sceneChangeDetection(@Nullable Boolean sceneChangeDetection) {
this.sceneChangeDetection = sceneChangeDetection;
return this;
}
@CustomType.Setter
public Builder stretchMode(@Nullable String stretchMode) {
this.stretchMode = stretchMode;
return this;
}
@CustomType.Setter
public Builder syncMode(@Nullable String syncMode) {
this.syncMode = syncMode;
return this;
}
public H264VideoResponse build() {
final var _resultValue = new H264VideoResponse();
_resultValue.complexity = complexity;
_resultValue.keyFrameInterval = keyFrameInterval;
_resultValue.label = label;
_resultValue.layers = layers;
_resultValue.odataType = odataType;
_resultValue.rateControlMode = rateControlMode;
_resultValue.sceneChangeDetection = sceneChangeDetection;
_resultValue.stretchMode = stretchMode;
_resultValue.syncMode = syncMode;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy