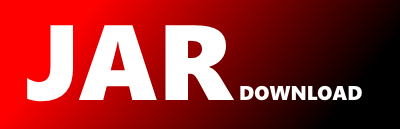
com.pulumi.azurenative.media.outputs.LiveEventInputResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.media.outputs;
import com.pulumi.azurenative.media.outputs.LiveEventEndpointResponse;
import com.pulumi.azurenative.media.outputs.LiveEventInputAccessControlResponse;
import com.pulumi.azurenative.media.outputs.LiveEventTimedMetadataEndpointResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class LiveEventInputResponse {
/**
* @return Access control for live event input.
*
*/
private @Nullable LiveEventInputAccessControlResponse accessControl;
/**
* @return A UUID in string form to uniquely identify the stream. This can be specified at creation time but cannot be updated. If omitted, the service will generate a unique value.
*
*/
private @Nullable String accessToken;
/**
* @return The input endpoints for the live event.
*
*/
private @Nullable List endpoints;
/**
* @return ISO 8601 time duration of the key frame interval duration of the input. This value sets the EXT-X-TARGETDURATION property in the HLS output. For example, use PT2S to indicate 2 seconds. Leave the value empty for encoding live events.
*
*/
private @Nullable String keyFrameIntervalDuration;
/**
* @return The input protocol for the live event. This is specified at creation time and cannot be updated.
*
*/
private String streamingProtocol;
/**
* @return The metadata endpoints for the live event.
*
*/
private @Nullable List timedMetadataEndpoints;
private LiveEventInputResponse() {}
/**
* @return Access control for live event input.
*
*/
public Optional accessControl() {
return Optional.ofNullable(this.accessControl);
}
/**
* @return A UUID in string form to uniquely identify the stream. This can be specified at creation time but cannot be updated. If omitted, the service will generate a unique value.
*
*/
public Optional accessToken() {
return Optional.ofNullable(this.accessToken);
}
/**
* @return The input endpoints for the live event.
*
*/
public List endpoints() {
return this.endpoints == null ? List.of() : this.endpoints;
}
/**
* @return ISO 8601 time duration of the key frame interval duration of the input. This value sets the EXT-X-TARGETDURATION property in the HLS output. For example, use PT2S to indicate 2 seconds. Leave the value empty for encoding live events.
*
*/
public Optional keyFrameIntervalDuration() {
return Optional.ofNullable(this.keyFrameIntervalDuration);
}
/**
* @return The input protocol for the live event. This is specified at creation time and cannot be updated.
*
*/
public String streamingProtocol() {
return this.streamingProtocol;
}
/**
* @return The metadata endpoints for the live event.
*
*/
public List timedMetadataEndpoints() {
return this.timedMetadataEndpoints == null ? List.of() : this.timedMetadataEndpoints;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(LiveEventInputResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable LiveEventInputAccessControlResponse accessControl;
private @Nullable String accessToken;
private @Nullable List endpoints;
private @Nullable String keyFrameIntervalDuration;
private String streamingProtocol;
private @Nullable List timedMetadataEndpoints;
public Builder() {}
public Builder(LiveEventInputResponse defaults) {
Objects.requireNonNull(defaults);
this.accessControl = defaults.accessControl;
this.accessToken = defaults.accessToken;
this.endpoints = defaults.endpoints;
this.keyFrameIntervalDuration = defaults.keyFrameIntervalDuration;
this.streamingProtocol = defaults.streamingProtocol;
this.timedMetadataEndpoints = defaults.timedMetadataEndpoints;
}
@CustomType.Setter
public Builder accessControl(@Nullable LiveEventInputAccessControlResponse accessControl) {
this.accessControl = accessControl;
return this;
}
@CustomType.Setter
public Builder accessToken(@Nullable String accessToken) {
this.accessToken = accessToken;
return this;
}
@CustomType.Setter
public Builder endpoints(@Nullable List endpoints) {
this.endpoints = endpoints;
return this;
}
public Builder endpoints(LiveEventEndpointResponse... endpoints) {
return endpoints(List.of(endpoints));
}
@CustomType.Setter
public Builder keyFrameIntervalDuration(@Nullable String keyFrameIntervalDuration) {
this.keyFrameIntervalDuration = keyFrameIntervalDuration;
return this;
}
@CustomType.Setter
public Builder streamingProtocol(String streamingProtocol) {
if (streamingProtocol == null) {
throw new MissingRequiredPropertyException("LiveEventInputResponse", "streamingProtocol");
}
this.streamingProtocol = streamingProtocol;
return this;
}
@CustomType.Setter
public Builder timedMetadataEndpoints(@Nullable List timedMetadataEndpoints) {
this.timedMetadataEndpoints = timedMetadataEndpoints;
return this;
}
public Builder timedMetadataEndpoints(LiveEventTimedMetadataEndpointResponse... timedMetadataEndpoints) {
return timedMetadataEndpoints(List.of(timedMetadataEndpoints));
}
public LiveEventInputResponse build() {
final var _resultValue = new LiveEventInputResponse();
_resultValue.accessControl = accessControl;
_resultValue.accessToken = accessToken;
_resultValue.endpoints = endpoints;
_resultValue.keyFrameIntervalDuration = keyFrameIntervalDuration;
_resultValue.streamingProtocol = streamingProtocol;
_resultValue.timedMetadataEndpoints = timedMetadataEndpoints;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy