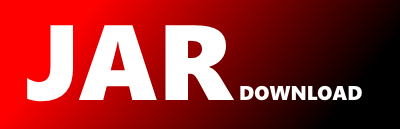
com.pulumi.azurenative.migrate.outputs.AKSAssessmentDetailsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.migrate.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class AKSAssessmentDetailsResponse {
/**
* @return Gets Confidence score.
*
*/
private Double confidenceRatingInPercentage;
/**
* @return Gets date and time when assessment was created.
*
*/
private String createdTimestamp;
/**
* @return Gets the number of machines.
*
*/
private Integer machineCount;
/**
* @return Gets last time when rates were queried.
*
*/
private String pricesTimestamp;
/**
* @return Gets assessment status.
*
*/
private String status;
/**
* @return Gets the total monthly cost.
*
*/
private Double totalMonthlyCost;
/**
* @return Gets date and time when assessment was last updated.
*
*/
private String updatedTimestamp;
/**
* @return Gets the number of web apps.
*
*/
private Integer webAppCount;
/**
* @return Gets the number of web servers.
*
*/
private Integer webServerCount;
private AKSAssessmentDetailsResponse() {}
/**
* @return Gets Confidence score.
*
*/
public Double confidenceRatingInPercentage() {
return this.confidenceRatingInPercentage;
}
/**
* @return Gets date and time when assessment was created.
*
*/
public String createdTimestamp() {
return this.createdTimestamp;
}
/**
* @return Gets the number of machines.
*
*/
public Integer machineCount() {
return this.machineCount;
}
/**
* @return Gets last time when rates were queried.
*
*/
public String pricesTimestamp() {
return this.pricesTimestamp;
}
/**
* @return Gets assessment status.
*
*/
public String status() {
return this.status;
}
/**
* @return Gets the total monthly cost.
*
*/
public Double totalMonthlyCost() {
return this.totalMonthlyCost;
}
/**
* @return Gets date and time when assessment was last updated.
*
*/
public String updatedTimestamp() {
return this.updatedTimestamp;
}
/**
* @return Gets the number of web apps.
*
*/
public Integer webAppCount() {
return this.webAppCount;
}
/**
* @return Gets the number of web servers.
*
*/
public Integer webServerCount() {
return this.webServerCount;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(AKSAssessmentDetailsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Double confidenceRatingInPercentage;
private String createdTimestamp;
private Integer machineCount;
private String pricesTimestamp;
private String status;
private Double totalMonthlyCost;
private String updatedTimestamp;
private Integer webAppCount;
private Integer webServerCount;
public Builder() {}
public Builder(AKSAssessmentDetailsResponse defaults) {
Objects.requireNonNull(defaults);
this.confidenceRatingInPercentage = defaults.confidenceRatingInPercentage;
this.createdTimestamp = defaults.createdTimestamp;
this.machineCount = defaults.machineCount;
this.pricesTimestamp = defaults.pricesTimestamp;
this.status = defaults.status;
this.totalMonthlyCost = defaults.totalMonthlyCost;
this.updatedTimestamp = defaults.updatedTimestamp;
this.webAppCount = defaults.webAppCount;
this.webServerCount = defaults.webServerCount;
}
@CustomType.Setter
public Builder confidenceRatingInPercentage(Double confidenceRatingInPercentage) {
if (confidenceRatingInPercentage == null) {
throw new MissingRequiredPropertyException("AKSAssessmentDetailsResponse", "confidenceRatingInPercentage");
}
this.confidenceRatingInPercentage = confidenceRatingInPercentage;
return this;
}
@CustomType.Setter
public Builder createdTimestamp(String createdTimestamp) {
if (createdTimestamp == null) {
throw new MissingRequiredPropertyException("AKSAssessmentDetailsResponse", "createdTimestamp");
}
this.createdTimestamp = createdTimestamp;
return this;
}
@CustomType.Setter
public Builder machineCount(Integer machineCount) {
if (machineCount == null) {
throw new MissingRequiredPropertyException("AKSAssessmentDetailsResponse", "machineCount");
}
this.machineCount = machineCount;
return this;
}
@CustomType.Setter
public Builder pricesTimestamp(String pricesTimestamp) {
if (pricesTimestamp == null) {
throw new MissingRequiredPropertyException("AKSAssessmentDetailsResponse", "pricesTimestamp");
}
this.pricesTimestamp = pricesTimestamp;
return this;
}
@CustomType.Setter
public Builder status(String status) {
if (status == null) {
throw new MissingRequiredPropertyException("AKSAssessmentDetailsResponse", "status");
}
this.status = status;
return this;
}
@CustomType.Setter
public Builder totalMonthlyCost(Double totalMonthlyCost) {
if (totalMonthlyCost == null) {
throw new MissingRequiredPropertyException("AKSAssessmentDetailsResponse", "totalMonthlyCost");
}
this.totalMonthlyCost = totalMonthlyCost;
return this;
}
@CustomType.Setter
public Builder updatedTimestamp(String updatedTimestamp) {
if (updatedTimestamp == null) {
throw new MissingRequiredPropertyException("AKSAssessmentDetailsResponse", "updatedTimestamp");
}
this.updatedTimestamp = updatedTimestamp;
return this;
}
@CustomType.Setter
public Builder webAppCount(Integer webAppCount) {
if (webAppCount == null) {
throw new MissingRequiredPropertyException("AKSAssessmentDetailsResponse", "webAppCount");
}
this.webAppCount = webAppCount;
return this;
}
@CustomType.Setter
public Builder webServerCount(Integer webServerCount) {
if (webServerCount == null) {
throw new MissingRequiredPropertyException("AKSAssessmentDetailsResponse", "webServerCount");
}
this.webServerCount = webServerCount;
return this;
}
public AKSAssessmentDetailsResponse build() {
final var _resultValue = new AKSAssessmentDetailsResponse();
_resultValue.confidenceRatingInPercentage = confidenceRatingInPercentage;
_resultValue.createdTimestamp = createdTimestamp;
_resultValue.machineCount = machineCount;
_resultValue.pricesTimestamp = pricesTimestamp;
_resultValue.status = status;
_resultValue.totalMonthlyCost = totalMonthlyCost;
_resultValue.updatedTimestamp = updatedTimestamp;
_resultValue.webAppCount = webAppCount;
_resultValue.webServerCount = webServerCount;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy