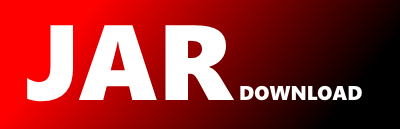
com.pulumi.azurenative.mobilenetwork.inputs.NaptConfigurationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.mobilenetwork.inputs;
import com.pulumi.azurenative.mobilenetwork.enums.NaptEnabled;
import com.pulumi.azurenative.mobilenetwork.inputs.PinholeTimeoutsArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.PortRangeArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.PortReuseHoldTimesArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The network address and port translation settings to use for the attached data network.
*
*/
public final class NaptConfigurationArgs extends com.pulumi.resources.ResourceArgs {
public static final NaptConfigurationArgs Empty = new NaptConfigurationArgs();
/**
* Whether NAPT is enabled for connections to this attached data network.
*
*/
@Import(name="enabled")
private @Nullable Output> enabled;
/**
* @return Whether NAPT is enabled for connections to this attached data network.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy