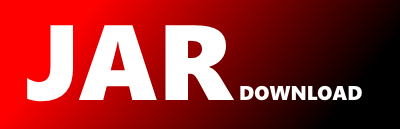
com.pulumi.azurenative.network.P2sVpnGatewayArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network;
import com.pulumi.azurenative.network.inputs.P2SConnectionConfigurationArgs;
import com.pulumi.azurenative.network.inputs.SubResourceArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class P2sVpnGatewayArgs extends com.pulumi.resources.ResourceArgs {
public static final P2sVpnGatewayArgs Empty = new P2sVpnGatewayArgs();
/**
* List of all customer specified DNS servers IP addresses.
*
*/
@Import(name="customDnsServers")
private @Nullable Output> customDnsServers;
/**
* @return List of all customer specified DNS servers IP addresses.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy