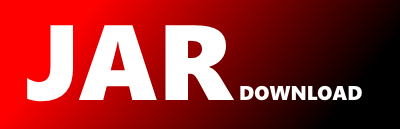
com.pulumi.azurenative.network.VirtualNetworkArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network;
import com.pulumi.azurenative.network.inputs.AddressSpaceArgs;
import com.pulumi.azurenative.network.inputs.DhcpOptionsArgs;
import com.pulumi.azurenative.network.inputs.ExtendedLocationArgs;
import com.pulumi.azurenative.network.inputs.SubResourceArgs;
import com.pulumi.azurenative.network.inputs.SubnetArgs;
import com.pulumi.azurenative.network.inputs.VirtualNetworkBgpCommunitiesArgs;
import com.pulumi.azurenative.network.inputs.VirtualNetworkEncryptionArgs;
import com.pulumi.azurenative.network.inputs.VirtualNetworkPeeringArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class VirtualNetworkArgs extends com.pulumi.resources.ResourceArgs {
public static final VirtualNetworkArgs Empty = new VirtualNetworkArgs();
/**
* The AddressSpace that contains an array of IP address ranges that can be used by subnets.
*
*/
@Import(name="addressSpace")
private @Nullable Output addressSpace;
/**
* @return The AddressSpace that contains an array of IP address ranges that can be used by subnets.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy